写一段earley代码
时间: 2024-12-18 22:20:23 浏览: 3
Earley算法是一种用于解析上下文无关文法(Context-Free Grammar, CFG)的自底向上方法。下面是简单的 Earley 算法的一个基本版本的 Python 示例,它实现了状态机的核心部分,用于处理文法规则的推导:
```python
class Item:
def __init__(self, symbol, index):
self.symbol = symbol
self.index = index
class EarleyParser:
def __init__(self, grammar, input_string):
self.items = []
self.productions = [(A, B) for A in grammar.start_symbols for B in grammar.productions[A]]
self.input_string = input_string
self.build()
def build(self):
for i in range(len(self.input_string)):
self.items.append(Item('$' + self.input_string[i], i))
def find_conflicts(self, state_set):
conflicts = set()
for item in state_set:
if item.is_complete():
production, dot_index = item.production, item.dot
# 检查当前项是否与其他已完成的项目冲突
conflict_item = None
for other_item in self.items:
if other_item.production == production and other_item.dot <= dot_index:
if conflict_item is not None:
conflicts.add((item, conflict_item))
else:
conflict_item = other_item
if conflict_item is not None:
conflicts.add((item, conflict_item))
return conflicts
def parse(self):
completed = {Item('$', -1)}
while completed:
new_items = set()
for item in completed:
if item not in self.items:
continue
if item.is_complete():
yield item.production
else:
for production, right_side in self.productions:
if item.symbol in right_side and len(right_side[item.symbol]) == item.dot:
new_items |= self.expand(item, production)
completed = new_items - completed
def expand(self, item, production):
expanded = set()
left_side, rest = production[:-1], production[-1]
if isinstance(rest, tuple): # 如果右部是一个终结符列表
for i in range(item.dot, len(left_side) + 1):
expanded.add(Item(left_side[:i] + (rest,), item.index))
else: # 如果右部是终结符
expanded.add(Item(left_side + (rest,), item.index))
return expanded
# 使用示例:
grammar = {'S': ['NP VP'],
'NP': [['Det N'], ['Det Adj N']],
'VP': [['V NP'], ['V Adv']]}
parser = EarleyParser(grammar, 'The quick brown fox')
for prod in parser.parse():
print(prod)
```
在这个例子中,`EarleyParser` 类维护了一个 `items` 列表,其中包含了所有可能的状态。`parse` 方法通过不断寻找新的完成状态并更新 `completed` 集合来驱动解析过程。
请注意,这只是一个简化的版本,实际应用中可能需要考虑更多的细节,如冲突解决策略、错误处理等。
阅读全文
相关推荐
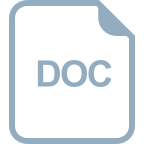
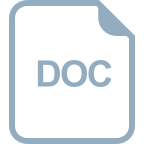
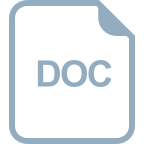
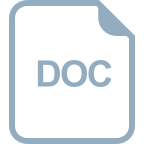
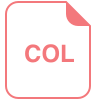

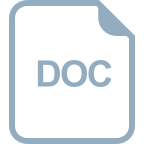
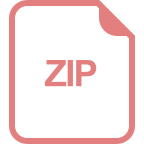
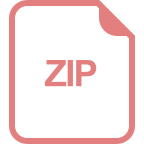
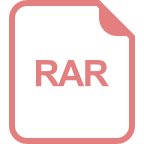
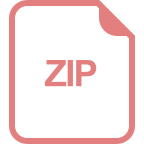
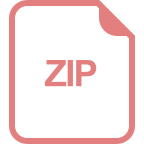
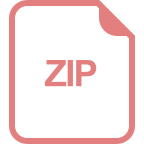
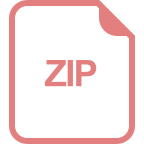
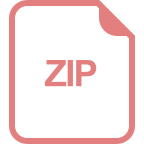