使用Python实现HTTP请求的三种方式(urllib模块、urllib3模块、requests模块),每种方式采用get和post两种请求模式,给出代码
时间: 2024-09-15 09:01:50 浏览: 42
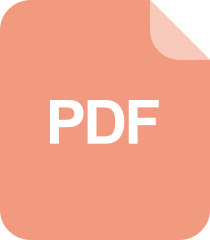
python使用urllib2提交http post请求的方法
在Python中,有几种常用的库用于发送HTTP请求,包括内置的`urllib`, `urllib3`, 和第三方库`requests`。这里我会分别演示如何使用这三种库来进行GET和POST请求:
1. **使用urllib模块** (基础版):
```python
import urllib.request
# GET请求
response_get = urllib.request.urlopen('http://example.com/api')
data_get = response_get.read().decode()
# POST请求 (使用urlencode)
from urllib.parse import urlencode
post_data = {'key': 'value'}
post_urlencoded = urlencode(post_data)
req_post = urllib.request.Request('http://example.com/api', post_urlencoded.encode())
with urllib.request.urlopen(req_post) as response_post:
post_data_response = response_post.read().decode()
```
2. **使用urllib3模块** (更高级,支持连接池等优化):
```python
import urllib3
http = urllib3.PoolManager()
# GET请求
response_get = http.request('GET', 'http://example.com/api')
data_get = response_get.data.decode()
# POST请求
post_data = {
'key': 'value'
}
headers = {'Content-Type': 'application/x-www-form-urlencoded'}
response_post = http.request('POST', 'http://example.com/api', fields=post_data, headers=headers)
post_data_response = response_post.data.decode()
```
3. **使用requests模块** (最常用,功能强大且易用):
```python
import requests
# GET请求
response_get = requests.get('http://example.com/api')
data_get = response_get.text
# POST请求
post_data = {'key': 'value'}
response_post = requests.post('http://example.com/api', data=post_data)
post_data_response = response_post.text
```
阅读全文
相关推荐
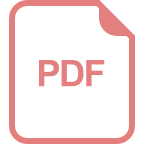
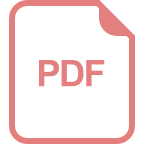



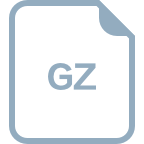
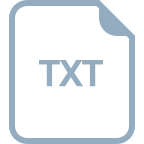
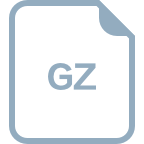
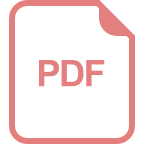
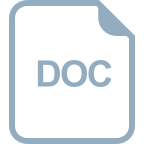
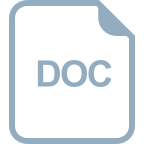
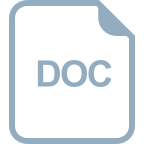
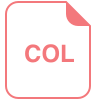
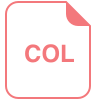
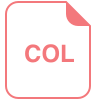


