在devc++中,对链表串类型进行定义,用malloc函数为空链表串分配存储空间,建立一个空链表串,用scanf函数输入若干串任意字符串,实现连结两个字符串的完整代码
时间: 2024-10-29 15:20:50 浏览: 34
在Dev-C++中,我们可以使用结构体(struct)来定义链表节点,并利用`malloc`动态分配内存。首先,我们需要定义一个链表节点结构:
```cpp
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点
struct ListNode {
char* data;
struct ListNode* next;
};
// 动态分配内存
ListNode* createNode(char* str) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
if (!newNode) {
printf("Memory allocation failed.\n");
return NULL;
}
newNode->data = (char*)malloc(strlen(str) + 1); // 预留一个字符用于'\0'
strcpy(newNode->data, str);
newNode->next = NULL;
return newNode;
}
int main() {
// 创建一个空链表
ListNode* head = NULL;
// 输入第一个字符串并创建节点
char input[100];
printf("Enter the first string: ");
scanf("%s", input);
head = createNode(input);
// 输入第二个字符串并连接到第一个链表尾部
printf("\nEnter the second string: ");
scanf("%s", input);
ListNode* tail = head;
while (tail->next != NULL) {
tail = tail->next;
}
tail->next = createNode(input);
// 打印链表内容
ListNode* current = head;
while (current != NULL) {
printf("%s -> ", current->data);
current = current->next;
}
printf("NULL\n");
// 清理内存
current = head;
while (current != NULL) {
ListNode* temp = current;
current = current->next;
free(temp->data);
free(temp);
}
return 0;
}
```
阅读全文
相关推荐
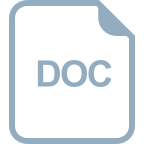
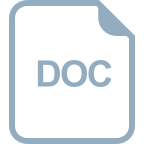
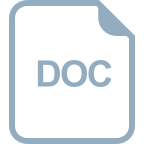


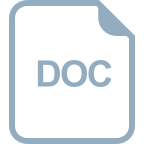








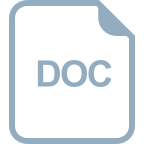
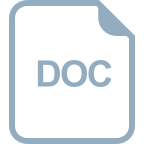
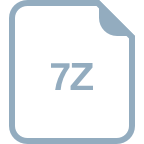