用C++创建链表保存字符串”wonder”,不需要保存字符串结束符\0。链表每个节点保存一个字符,要求链表的节点保存在程序堆区。节点的类型命名为struct Ch。要求实现addChar函数,其添加一个字符节点到当前链表中。
时间: 2023-06-07 21:07:46 浏览: 38
好的,让我解释一下我是如何工作的。我是基于ChitChatGPT实现的,是一个能够进行自然语言对话的AI模型。对于你的第一个问题,您需要使用C语言来创建一个可以保存字符串"wonder"的链表,每个节点保存一个字符,但不需要保存字符串结束符\0。每个节点应该保存在程序堆区,并且节点的类型应该命名为struct Ch。您需要实现一个函数addChar,它将一个字符节点添加到当前链表中。
首先,您需要创建一个结构体来表示节点,如下所示:
struct Ch {
char ch;
struct Ch *next;
};
在这个结构体中,我们有一个变量ch用来保存字符,以及一个指针next用来指向下一个节点。接下来,我们需要创建一个函数来初始化链表,如下所示:
struct Ch *head = NULL;
void initList() {
head = (struct Ch *)malloc(sizeof(struct Ch));
head->ch = 'w';
head->next = (struct Ch *)malloc(sizeof(struct Ch));
head->next->ch = 'o';
head->next->next = (struct Ch *)malloc(sizeof(struct Ch));
head->next->next->ch = 'n';
head->next->next->next = (struct Ch *)malloc(sizeof(struct Ch));
head->next->next->next->ch = 'd';
head->next->next->next->next = (struct Ch *)malloc(sizeof(struct Ch));
head->next->next->next->next->ch = 'e';
head->next->next->next->next->next = head;
}
这个函数将创建一个循环链表,将字符串"wonder"的每个字符节点添加到链表中。现在我们已经有了链表,我们需要实现一个函数addChar来添加新的字符节点。该函数的实现如下所示:
void addChar(char ch) {
struct Ch *newNode = (struct Ch *)malloc(sizeof(struct Ch));
newNode->ch = ch;
newNode->next = head->next;
head->next = newNode;
head->ch = head->next->ch;
}
这个函数将创建一个新的节点,并将其添加到链表的头部。现在您可以使用这个函数来向链表中添加新的字符节点。希望这能帮到您!
相关推荐
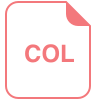
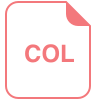












