将字符串输入链表,其中每个节点只保存一个字符
时间: 2024-05-12 07:19:47 浏览: 107
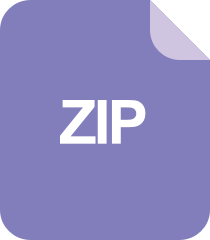
字符串插入块链实现.zip_块链 串_字符串_链块插入_链表
可以使用链表的基本操作,如创建节点、插入节点和遍历链表等。具体步骤如下:
1. 定义链表节点结构体,包含一个字符类型的数据和一个指向下一个节点的指针。
```
struct ListNode {
char val;
ListNode *next;
ListNode(char x) : val(x), next(NULL) {}
};
```
2. 创建一个头结点,表示链表的开始。
```
ListNode* head = new ListNode(-1); // 头结点
ListNode* cur = head; // 当前节点
```
3. 遍历字符串中的每个字符,创建一个节点并插入链表。
```
string s = "hello";
for (int i = 0; i < s.size(); i++) {
ListNode* node = new ListNode(s[i]); // 创建节点
cur->next = node; // 插入节点
cur = cur->next; // 更新当前节点
}
```
4. 遍历链表,输出每个节点的值。
```
cur = head->next; // 从第一个节点开始遍历
while (cur != NULL) {
cout << cur->val << " "; // 输出节点值
cur = cur->next; // 更新当前节点
}
```
完整代码如下:
```
#include <iostream>
#include <string>
using namespace std;
struct ListNode {
char val;
ListNode *next;
ListNode(char x) : val(x), next(NULL) {}
};
int main() {
ListNode* head = new ListNode(-1); // 头结点
ListNode* cur = head; // 当前节点
string s = "hello";
for (int i = 0; i < s.size(); i++) {
ListNode* node = new ListNode(s[i]); // 创建节点
cur->next = node; // 插入节点
cur = cur->next; // 更新当前节点
}
cur = head->next; // 从第一个节点开始遍历
while (cur != NULL) {
cout << cur->val << " "; // 输出节点值
cur = cur->next; // 更新当前节点
}
return 0;
}
```
阅读全文
相关推荐
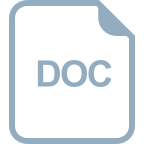
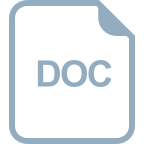
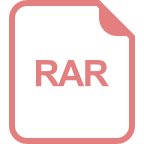
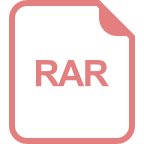
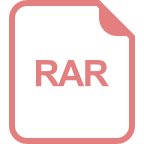
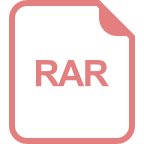
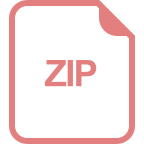
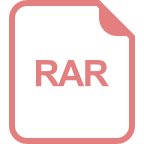
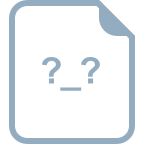
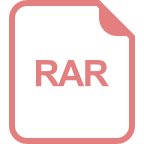
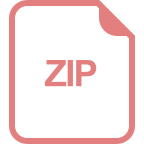
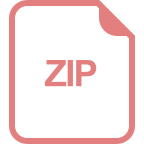
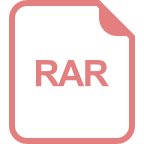
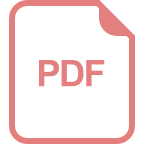
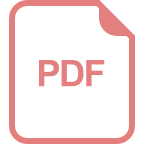
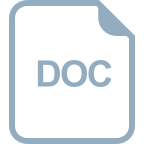
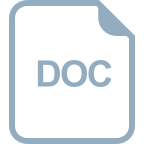