C语言中链表与字符串之间的关联及应用场景
发布时间: 2024-03-30 20:38:54 阅读量: 65 订阅数: 26 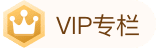
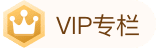
# 1. 理解链表和字符串
链表和字符串在C语言中都是非常重要的数据结构,它们在程序设计中具有各自独特的特点和应用场景。在本章中,我们将深入理解链表和字符串的概念以及它们在C语言中的表示方法。
# 2. 链表与字符串的相似之处
链表和字符串在某些方面存在相似之处,下面将分别探讨它们之间的相似之处。
### 2.1 结构化存储数据
链表是一种线性数据结构,由节点组成,每个节点包含数据和指向下一个节点的指针。类似地,字符串也是一种线性数据结构,由字符序列组成。链表中的节点可以看做是存储字符的单元,从而可以结构化地存储字符串数据。
### 2.2 动态分配内存
链表和字符串在内存管理方面都具有动态性。链表节点的动态分配和释放可以在运行时进行,灵活性很高。字符串在C语言中本质上是以字符数组的形式存在,也可以通过动态分配内存来操作,动态变化字符串的长度。
### 2.3 遍历和操作元素
在处理数据时,链表和字符串都可以遍历各个元素进行操作。链表可以通过遍历节点来访问、修改数据,而字符串也可以逐个访问字符进行处理,比如统计字符个数、串联字符串等操作。链表和字符串的遍历操作在处理数据时起着至关重要的作用。
链表和字符串之间的相似之处使得它们可以在某些场景下相互转换、结合使用,为数据处理提供更多的选择和灵活性。
# 3. 链表中存储字符串的实现方法
在C语言中,链表可以被用来存储字符串数据。这样的设计在某些情况下可以提供更灵活的操作和管理方式。下面我们将介绍链表中存储字符串的几种实现方法:
#### 3.1 单链表存储字符串
单链表中每个结点可以存储一个字符,通过链接结点形成字符串。这种方法的优点是节省空间,但在字符串操作和查找上效率较低。
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
char data;
struct Node* next;
};
// 函数用于打印输出单链表中存储的字符串
void printString(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%c", current->data);
current = current->next;
}
}
int main() {
struct Node* head = NULL;
struct Node* second = NULL;
struct Node* third = NULL;
head = (struct Node*)malloc(sizeof(struct Node));
second = (struct Node*)malloc(sizeof(struct Node));
third = (struct Node*)malloc(sizeof(struct Node));
head->data = 'H';
head->next = second;
second->data = 'e';
second->next = third;
third->data = 'l';
third->next = NULL;
printString(head);
return 0;
}
```
**代码总结:** 这段代码通过单链表存储了一个字符串"Hel"并打印输出。
**结果说明:** 运行代码将输出"Hel"。
#### 3.2 双链表存储字符串
双链表中每个结点除了包含数据外,还有指向前后结点的指针,能够更快速地进行字符串的操作和查找。
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
char data;
struct Node* prev;
struct Node* next;
};
// 函数用于打印输出双链表中存储的字符串
void printString(struct Node* head) {
struct Node* current = head;
while (current != NULL) {
printf("%c", current->data);
current = current->next;
}
}
int main() {
struct Node* head = NULL;
struct Node* second = NULL;
struct Node* third = NULL;
head = (struct Node*)malloc(sizeof(struct Node));
second = (struct Node*)malloc(sizeof(struct Node));
thi
```
0
0
相关推荐
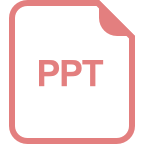
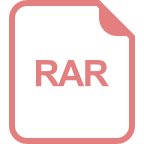
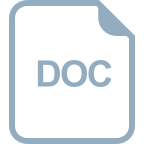
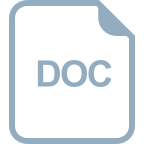
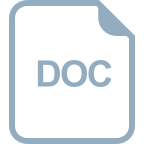
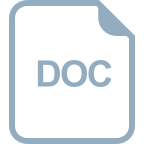
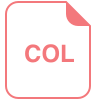