写一个双向链表逆向输出字符串c语言
时间: 2023-09-10 07:12:39 浏览: 119
以下是一个简单的双向链表逆向输出字符串的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 双向链表结构体
typedef struct node {
char data;
struct node* prev;
struct node* next;
} Node;
// 创建双向链表
Node* createList(const char* str) {
Node* head = NULL;
Node* tail = NULL;
int len = strlen(str);
for (int i = 0; i < len; i++) {
Node* node = (Node*)malloc(sizeof(Node));
node->data = str[i];
node->prev = tail;
node->next = NULL;
if (tail) {
tail->next = node;
} else {
head = node;
}
tail = node;
}
return head;
}
// 释放双向链表内存
void freeList(Node* head) {
Node* node = head;
while (node) {
Node* next = node->next;
free(node);
node = next;
}
}
// 逆向输出双向链表
void reversePrint(Node* tail) {
Node* node = tail;
while (node) {
printf("%c", node->data);
node = node->prev;
}
}
int main() {
const char* str = "Hello, world!";
Node* head = createList(str);
reversePrint(head);
freeList(head);
return 0;
}
```
在这个代码中,我们首先使用 `createList` 函数创建了一个双向链表,然后使用 `reversePrint` 函数逆向输出了链表中的字符。最后,我们使用 `freeList` 函数释放了双向链表的内存。
阅读全文
相关推荐
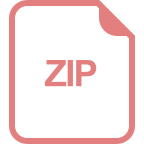
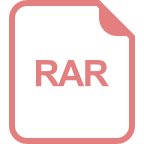
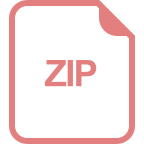
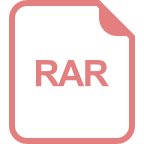
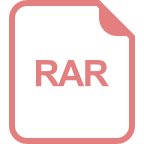
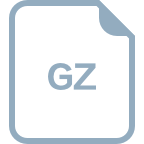
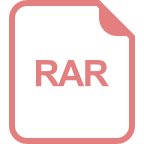
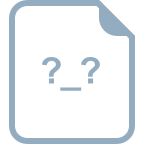
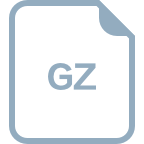
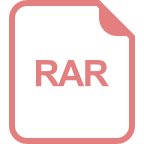
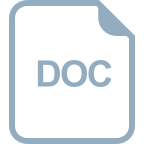
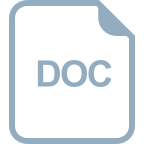