带有混合时间窗多配送中心多目标车辆路径优化粒子群算法初始化部分代码
时间: 2023-09-15 20:19:49 浏览: 105
以下是带有混合时间窗多配送中心多目标车辆路径优化的粒子群算法初始化部分的示例代码:
```python
import numpy as np
class Particle:
def __init__(self, num_vehicles, num_customers, num_depots):
self.num_vehicles = num_vehicles
self.num_customers = num_customers
self.num_depots = num_depots
self.position = np.zeros((num_vehicles, num_customers+1), dtype=int)
self.velocity = np.zeros((num_vehicles, num_customers+1), dtype=int)
self.best_position = self.position.copy()
self.best_fitness = float('inf')
class PSO:
def __init__(self, num_particles, num_vehicles, num_customers, num_depots):
self.num_particles = num_particles
self.num_vehicles = num_vehicles
self.num_customers = num_customers
self.num_depots = num_depots
self.particles = []
self.global_best_fitness = float('inf')
self.global_best_position = None
def initialize_particles(self):
for _ in range(self.num_particles):
particle = Particle(self.num_vehicles, self.num_customers, self.num_depots)
for i in range(self.num_vehicles):
# Generate random route for each vehicle
customer_indices = np.arange(1, self.num_customers+1)
np.random.shuffle(customer_indices)
particle.position[i, 1:] = customer_indices
particle.position[:, 0] = np.random.randint(low=0, high=self.num_depots, size=self.num_vehicles)
particle.best_position = particle.position.copy()
self.particles.append(particle)
# Rest of the PSO code...
if __name__ == '__main__':
num_particles = 50
num_vehicles = 3
num_customers = 10
num_depots = 2
pso = PSO(num_particles, num_vehicles, num_customers, num_depots)
pso.initialize_particles()
```
以上代码示例演示了如何初始化粒子群算法的粒子。在 `initialize_particles` 方法中,每个粒子的位置 `position` 是一个二维数组,其中每行表示一个车辆的路径,第一列是车辆的起始位置(配送中心),后续列是顾客的访问顺序。
在示例代码中,我们使用了随机生成的初始路径。根据您的具体问题,您可能需要根据一些启发式规则或其他算法生成更合适的初始路径。请根据您的问题需求进行相应的修改。
如果您有其他问题,请随时提问!
阅读全文
相关推荐
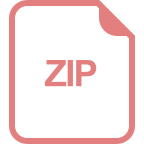
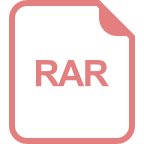
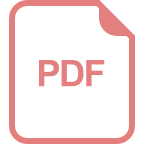
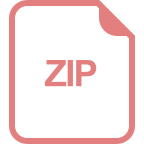
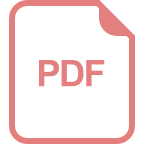
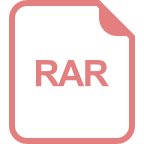
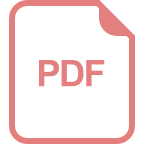
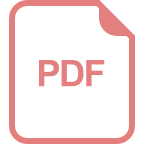
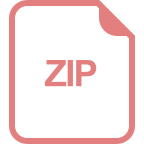
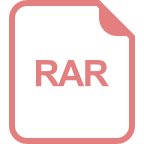
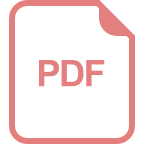
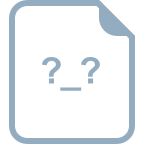
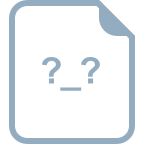
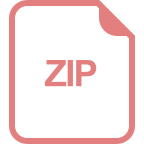
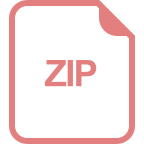