请求提供用C语言编写的三种常见页面置换算法(如LRU、FIFO和Optimal)的完整代码实现示例。
时间: 2024-12-13 09:22:33 浏览: 132
在C语言中,我们可以用结构体和链表来实现一些常见的页面置换算法。以下是三个例子:LRU (最近最少使用),FIFO (先进先出) 和 Optimal (最佳适应)。这里只给出基本的伪代码和关键部分,因为完整的实现会涉及内存管理的细节。
1. LRU (Least Recently Used):
```c
typedef struct Node {
int data;
struct Node* next;
struct Node* prev;
} Node;
Node* head = NULL;
Node* tail = NULL;
void insert(int value) {
// ... 实现插入并调整头尾指针
}
// 模拟LRU替换操作,这里简化了删除和移动操作
void evict() {
if (!head) return;
Node* temp = head;
head = head->next; // 删除最旧的节点
free(temp);
}
```
2. FIFO (First In First Out):
```c
struct Page {
int data;
struct Page* next;
};
Page* first = NULL;
Page* last = NULL;
void insert(int value) {
// ... 创建新页并添加到列表末尾
}
void evict() {
if (!first) return;
Page* temp = first;
first = first->next;
free(temp);
}
```
3. Optimal (Best Fit):
```c
// 这里假设有一个空闲块列表FreePages
// 以及一个函数findFit()用于查找最小的合适大小的空闲块
void insert(int value) {
// ... 找到最小的空闲块,调整数据和引用
findFit(value); // 省略具体的搜索和分配代码
}
void evict() {
// ... 需要遍历FreePages找到最小的页面替换,这需要额外的数据结构支持
}
```
阅读全文
相关推荐
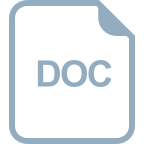
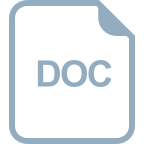
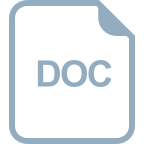
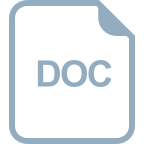


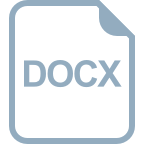
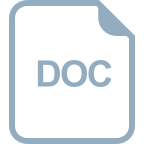
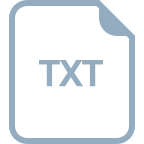
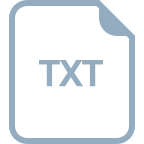
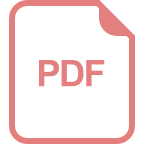
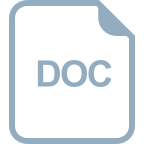




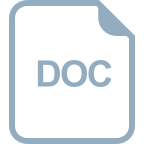
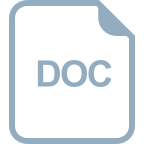
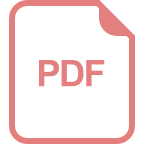