求解一般集合的并集问题。 已知两个集合a和b,现要求一个新的集合a=aub。例如,设 a=(7,5,3,11) b=(2,6,3) 合并后 a=(7,5,3,11,2,6
时间: 2024-10-11 07:07:39 浏览: 80
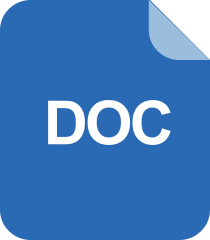
高一数学上学期第一次月考试题(无答案)苏教版 试题.doc
在C#中,你可以使用`HashSet<T>`或`List<T>`等数据结构来求解集合的并集。这里我们使用`HashSet`因为它的插入查找操作都具有较好的时间复杂度。如果你的元素没有重复,`HashSet`将是理想的选择。如果可能有重复,`List<T>`加上去重操作也是可行的。
下面是用`HashSet`求解的例子:
```csharp
using System;
using System.Collections.Generic;
class Program
{
static void Main(string[] args)
{
// 定义初始集合
HashSet<int> setA = new HashSet<int>() { 7, 5, 3, 11 };
HashSet<int> setB = new HashSet<int>() { 2, 6, 3 };
// 使用UnionWith方法计算并集
setA.UnionWith(setB);
// 输出结果
foreach (int item in setA)
{
Console.Write(item + " ");
}
}
}
```
运行这段代码,输出将会是 `7 5 3 11 2 6`,这就是集合a和b的并集。
如果你想使用列表并且包含重复元素,可以这样做:
```csharp
List<int> listA = new List<int>() { 7, 5, 3, 11 };
List<int> listB = new List<int>() { 2, 6, 3 };
// 将setB转换为list,然后合并
listA.AddRange(listB.Distinct());
foreach (int item in listA)
{
Console.Write(item + " ");
}
```
在这里,`Distinct()` 方法用于去除重复项。
阅读全文
相关推荐
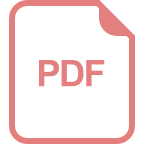
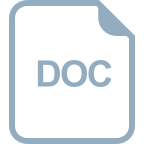


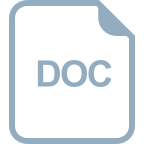
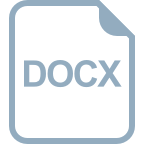
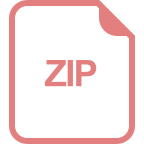
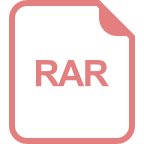
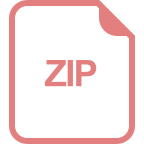
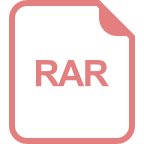
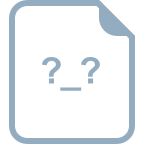
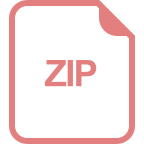
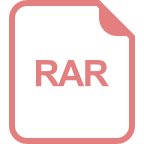
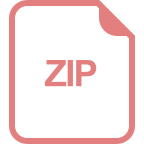