ubus数据解析多层嵌套BLOBMSG_TYPE_TABLE或BLOBMSG_TYPE_ARRAY,代码示例
时间: 2023-08-07 09:08:39 浏览: 232
当ubus数据具有多层嵌套的BLOBMSG_TYPE_TABLE或BLOBMSG_TYPE_ARRAY类型时,可以使用递归的方式进行解析。下面是一个示例代码,演示了如何解析多层嵌套的ubus数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <libubox/blobmsg_json.h>
void parse_blobmsg_table(const struct blob_attr *attr);
void parse_blobmsg_array(const struct blob_attr *attr)
{
int rem;
struct blob_attr *tb[BLOBMSG_TYPE_MAX + 1];
struct blob_attr *cur;
blobmsg_for_each_attr(cur, attr, rem)
{
if (blobmsg_type(cur) == BLOBMSG_TYPE_TABLE)
{
parse_blobmsg_table(cur);
}
else if (blobmsg_type(cur) == BLOBMSG_TYPE_ARRAY)
{
parse_blobmsg_array(cur);
}
else
{
fprintf(stderr, "Unexpected blobmsg type\n");
}
}
}
void parse_blobmsg_table(const struct blob_attr *attr)
{
int rem;
struct blob_attr *tb[BLOBMSG_TYPE_MAX + 1];
struct blob_attr *cur;
blobmsg_parse(tb, BLOBMSG_TYPE_MAX, blobmsg_data(attr), blobmsg_data_len(attr));
if (!tb[0])
{
fprintf(stderr, "Failed to parse blobmsg table\n");
return;
}
blobmsg_for_each_attr(cur, tb[0], rem)
{
if (blobmsg_type(cur) == BLOBMSG_TYPE_TABLE)
{
parse_blobmsg_table(cur);
}
else if (blobmsg_type(cur) == BLOBMSG_TYPE_ARRAY)
{
parse_blobmsg_array(cur);
}
else
{
// Handle individual values
// You can access the value using blobmsg_get_type() and blobmsg_get_* functions
const char *key = blobmsg_name(cur);
int val = blobmsg_get_u32(cur);
printf("Key: %s, Value: %d\n", key, val);
}
}
}
int main()
{
// Example ubus data in JSON format
const char *json_data = "{\"table\":{\"nested_table\":{\"array\":[1,2,3]},\"value\":123}}";
struct blob_attr *attr;
struct blob_buf buf;
blob_buf_init(&buf, 0);
if (blobmsg_add_json_from_string(&buf, json_data) != 0)
{
fprintf(stderr, "Failed to parse JSON data\n");
return 1;
}
attr = buf.head;
if (!attr)
{
fprintf(stderr, "Empty blobmsg data\n");
return 1;
}
parse_blobmsg_table(attr);
return 0;
}
```
在这个示例代码中,我们使用了libubox库中的函数来解析ubus数据。首先,我们将JSON格式的ubus数据转换为blobmsg格式,然后使用parse_blobmsg_table函数进行解析。在解析过程中,如果遇到嵌套的BLOBMSG_TYPE_TABLE类型或BLOBMSG_TYPE_ARRAY类型,就会递归调用相应的解析函数。
在parse_blobmsg_table函数中,我们使用了blobmsg_type和blobmsg_for_each_attr函数来遍历解析后的数据。对于嵌套的BLOBMSG_TYPE_TABLE和BLOBMSG_TYPE_ARRAY类型,我们会分别调用parse_blobmsg_table和parse_blobmsg_array函数进行解析。对于其他类型,我们可以使用blobmsg_get_*函数来获取具体的值。
请注意,这只是一个简单的示例代码,实际的解析过程可能更加复杂,需要根据具体的ubus数据结构和需求进行适当的调整。
相关推荐
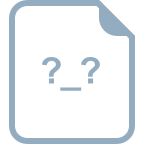














