c语言循环语句延时方法
时间: 2024-06-22 18:01:57 浏览: 91
在C语言中,实现循环语句的延迟(暂停执行)通常使用`sleep()`函数,它来自于头文件`<unistd.h>`。这个函数用于让当前进程睡眠指定的秒数。例如,如果你想让程序暂停一秒,你可以这样做:
```c
#include <unistd.h>
#include <sys/time.h> // 需要这个头文件来使用time_t类型
// 假设你需要延迟1秒钟
#define DELAY_SECONDS 1
void delay(int seconds) {
struct timespec ts;
ts.tv_sec = seconds; // 设置秒数
ts.tv_nsec = 0; // 如果需要毫秒级别的精度,设置毫秒数
nanosleep(&ts, NULL); // 实行睡眠
}
int main() {
for (int i = 0; i < 10; i++) {
printf("Iteration %d\n", i);
delay(DELAY_SECONDS); // 在每次迭代后暂停
}
return 0;
}
```
相关问题
C语言空语句循环延时50微秒
C语言中的空语句循环用于实现延时。下面是实现50微秒延时的代码:
```
#include <time.h>
void delay(int microseconds)
{
clock_t start = clock();
while (clock() - start < microseconds)
;
}
int main()
{
delay(50);
return 0;
}
```
请注意,这种方法不是精确的延时,它受到系统性能的影响。如果需要精确的延时,建议使用系统特定的函数,如 `usleep` 或 `nanosleep`。
C语言用for语句延时一秒
可以使用以下代码:
```c
#include <stdio.h>
#include <time.h>
void delay(int seconds) {
int end = clock() + seconds * CLOCKS_PER_SEC;
while(clock() < end);
}
int main() {
printf("Start\n");
delay(1);
printf("End\n");
return 0;
}
```
在这段代码中,使用了 `clock()` 函数来获取当前 CPU 时间,并且将其转换成每秒的 clock 值。然后,使用一个 while 循环来进行延时,直到当前时间超过了指定的时间。在主函数中,我们调用 `delay(1)` 函数来延时一秒,并在开始和结束时打印出一些文本以便查看程序的运行过程。
阅读全文
相关推荐
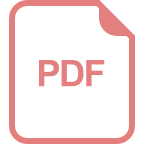
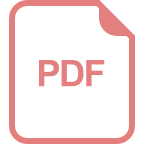
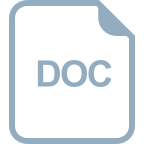
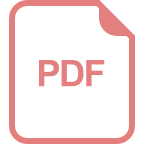
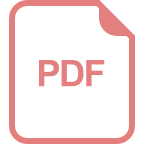
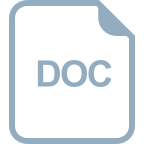
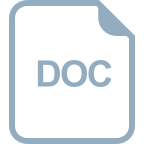
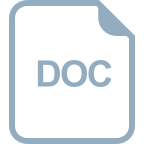
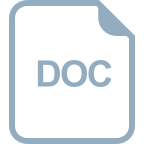
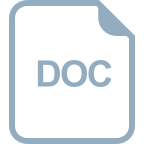
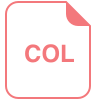
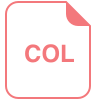
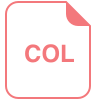
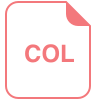