在Hadoop分布式文件系统(HDFS)上使用Java实现MapReduce进行WordCount词频统计时,如何构建Mapper和Reducer的代码逻辑,并配置MapReduce作业的参数?
时间: 2024-10-30 17:19:45 浏览: 24
为了实现基于HDFS的MapReduce WordCount词频统计,你需要编写特定的MapReduce作业,其中包含Mapper和Reducer两个主要组件。以下是一个具体实现的示例,以及如何配置作业参数的详细步骤。
参考资源链接:[MapReduce实战:WordCount词频统计与HDFS应用](https://wenku.csdn.net/doc/4czhvwub77?spm=1055.2569.3001.10343)
首先,你需要准备Hadoop开发环境,并确保HDFS服务已经启动。以下是实现WordCount的步骤和代码细节:
1. **编写Mapper类**:Mapper负责读取输入的文本行,并将每个单词映射为键值对(word, 1)。关键在于如何分割文本并生成中间键值对。
```java
public static class TokenizerMapper extends Mapper<Object, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(Object key, Text value, Context context) throws IOException, InterruptedException {
StringTokenizer itr = new StringTokenizer(value.toString());
while (itr.hasMoreTokens()) {
word.set(itr.nextToken());
context.write(word, one);
}
}
}
```
2. **编写Reducer类**:Reducer负责对相同单词的键值对进行合并,即对所有计数求和,得到每个单词的频率。
```java
public static class IntSumReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
private IntWritable result = new IntWritable();
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
result.set(sum);
context.write(key, result);
}
}
```
3. **配置和运行MapReduce作业**:在主函数中配置作业的输入输出路径,设置Mapper和Reducer类,以及其他作业参数。然后提交作业并等待完成。
```java
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf,
参考资源链接:[MapReduce实战:WordCount词频统计与HDFS应用](https://wenku.csdn.net/doc/4czhvwub77?spm=1055.2569.3001.10343)
阅读全文
相关推荐
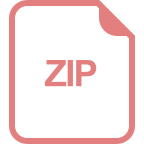
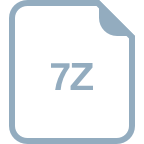
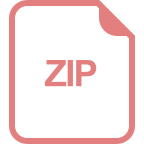
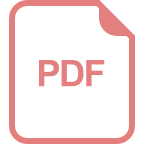
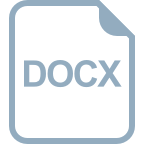
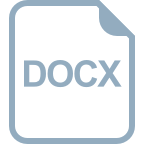
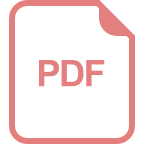
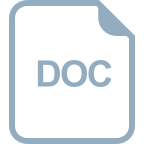
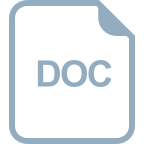
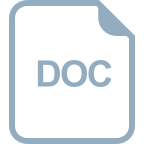
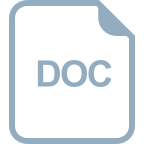
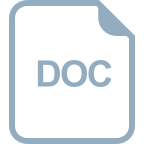
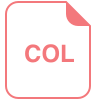
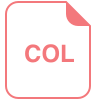
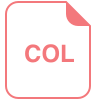



