drawable对象转成mipmap
时间: 2023-08-04 11:05:16 浏览: 47
要将 `drawable` 对象转换为 `mipmap`,你可以按照以下步骤进行操作:
1. 首先,确保你的项目中存在 `mipmap` 目录。如果不存在,可以在 `res` 目录下创建一个名为 `mipmap` 的新目录。
2. 将你的 `drawable` 资源文件复制到 `mipmap` 目录下。你可以直接将文件复制到 `mipmap` 目录中,或者通过拖放操作将文件移动到 `mipmap` 目录。
3. 在布局文件或代码中使用 `mipmap` 资源。与使用 `drawable` 资源相似,你可以使用 `@mipmap/` 前缀来引用 `mipmap` 资源。例如,如果你有一个名为 `my_image.png` 的图像资源,你可以在布局文件中使用以下代码引用它:
```xml
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@mipmap/my_image"
/>
```
请记住,`mipmap` 目录主要用于应用程序的图标资源,而不是通用的图片资源。在大多数情况下,你应该将其他图片资源放在 `drawable` 目录中。
相关问题
安卓实现下载图片到mipmap
安卓开发中,我们可以通过代码实现将图片下载到mipmap中。
首先,我们需要创建一个AsyncTask类用于在后台下载图片,该类需要三个泛型参数,分别是参数类型,进度类型和结果类型。
接着,在AsyncTask类的doInBackground方法中,我们可以使用HttpURLConnection或OkHttpClient等网络库发送网络请求,将图片下载到本地。
最后,我们可以在AsyncTask类的onPostExecute方法中获取下载的结果,并将图片保存到mipmap中。
具体步骤如下:
1. 创建一个AsyncTask类,用于在后台下载图片。
```
class DownloadTask extends AsyncTask<String, Void, Bitmap> {
@Override
protected Bitmap doInBackground(String... urls) {
String imageUrl = urls[0];
Bitmap bitmap = null;
try {
URL url = new URL(imageUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoInput(true);
conn.connect();
InputStream inputStream = conn.getInputStream();
bitmap = BitmapFactory.decodeStream(inputStream);
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
return bitmap;
}
@Override
protected void onPostExecute(Bitmap result) {
if(result != null){
addToMipmap(result);
}
}
}
```
2. 在AsyncTask类的doInBackground方法中,使用HttpURLConnection或OkHttpClient等网络库发送网络请求,将图片下载到本地。
```
URL url = new URL(imageUrl);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setDoInput(true);
conn.connect();
InputStream inputStream = conn.getInputStream();
bitmap = BitmapFactory.decodeStream(inputStream);
inputStream.close();
```
3. 在AsyncTask类的onPostExecute方法中,获取下载的结果,并将图片保存到mipmap中。
```
private void addToMipmap(Bitmap bitmap) {
try {
Resources res = getResources();
String imageName = "downloaded_image";
int drawableId = res.getIdentifier(imageName, "mipmap", getPackageName());
Drawable drawable = new BitmapDrawable(res, bitmap);
((BitmapDrawable) drawable).setGravity(Gravity.CENTER);
if (drawableId != 0) {
res.getDrawable(drawableId);
}
} catch (Exception e) {
e.printStackTrace();
}
}
```
在addToMipmap方法中,我们通过调用getResources方法获取资源对象,使用getIdentifier方法获取mipmap中的资源ID,然后将下载的图片转化成Drawable对象,并将其保存到mipmap中。
最后,在Activity中,我们可以调用DownloadTask类的execute方法,启动图片下载任务。
```
new DownloadTask().execute(imageUrl);
```
MainActivity3对应activity_main3.xml,imageView在item.xml,id为imageView2。在MainActivity3中运行imageView=findViewById(R.id.imageView2);imageView.setImageResource(R.mipmap.blue);出现空指针异常
空指针异常通常是因为对象没有被正确地初始化。根据你提供的信息,可能是以下几种情况导致的:
1. item.xml 中没有定义 id 为 imageView2 的 ImageView。请确保 item.xml 中有一个 ImageView,它的 id 属性设置为 imageView2。
2. MainActivity3 中没有使用 setContentView 方法加载 activity_main3.xml。请确保 MainActivity3 中使用 setContentView 方法加载 activity_main3.xml,否则 findViewById 方法无法找到对应的控件。
3. R.mipmap.blue 图片不存在。请确保 R.mipmap.blue 表示的图片存在于 drawable 目录下。
请检查以上几点,如果仍然无法解决问题,请提供更多信息,以便我能够更准确地判断问题所在。
相关推荐







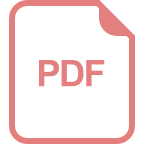
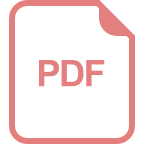
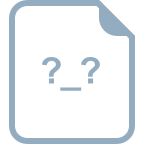
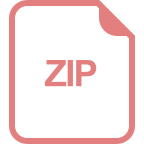