如何用python写出一个乒乓球小游戏,用到turtle,并且有单人双人两种模式
时间: 2024-10-11 20:11:22 浏览: 27
要创建一个简单的乒乓球游戏,我们可以使用Python的`turtle`库,结合基本的游戏逻辑和用户交互。这里我会提供一个基础版本的代码,包括单人模式(玩家对电脑)和双人模式(两个玩家互相对战)。请注意,这个示例不会包含完整的图形界面,因为`turtle`主要用于绘图,而不适合复杂的事件处理。你可以根据需要扩展和完善。
首先,确保已经安装了`turtle`库,如果没有,可以通过pip进行安装:
```bash
pip install turtle
```
接下来,我们编写一个基本框架:
```python
import turtle
import random
# 设置屏幕大小和初始位置
window = turtle.Screen()
window.bgcolor("black")
player1 = turtle.Turtle()
player1.speed(0)
player1.shape("square") # 更改形状为方块
player1.penup()
player1.goto(-350, 0)
computer_player = turtle.Turtle()
computer_player.speed(0)
computer_player.shape("circle")
computer_player.penup()
computer_player.goto(350, 0)
ball = turtle.Turtle()
ball.shape("circle")
ball.color("white")
ball.penup()
ball.goto(0, 0)
ball.dx = random.choice([-3, -2, -1, 1, 2, 3]) # 初始球速随机
ball.dy = random.randint(-3, 3) # 初始球上下移动速度范围
def serve():
ball.goto(0, 280)
ball.dy *= -1
def paddle_move(event):
if event.x > player1.xcor() and event.x < player1.xcor() + 50:
player1.setx(event.x)
def computer_move():
global ball
angle = (ball.ycor() + 150) / 280 * 90
computer_player.setheading(angle)
# 设置事件监听器
window.onkey(paddle_move, "w")
window.onkey(paddle_move, "s")
serve()
while True:
window.update()
ball.sety(ball.ycor() + ball.dy)
ball.setx(ball.xcor() + ball.dx)
# 检查边界碰撞
if ball.ycor() > 270 or ball.ycor() < -270:
ball.dy *= -1
if ball.distance(player1) < 50 or ball.distance(computer_player) < 50:
ball.dx *= -1
ball.dy *= -1
serve()
# 计算并执行计算机玩家的动作
if random.random() < 0.1:
computer_move()
window.listen()
window.mainloop()
```
这个代码实现了以下功能:
- 单人模式:玩家控制方块移动来接住从屏幕顶部反弹的球。
- 双人模式:玩家1和2分别通过键盘上的上、下箭头键控制自己的小方块。
- 电脑玩家偶尔会自动调整角度击球,模拟更真实的比赛。
为了使游戏更加完整,你可以添加得分系统,计时,以及当一方达到特定分数后结束游戏的功能。此外,可能还需要优化碰撞检测算法和增加游戏规则。
阅读全文
相关推荐
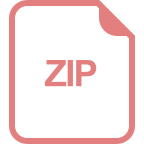
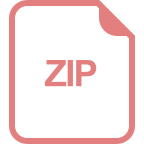
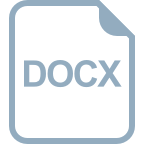
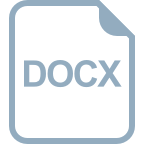

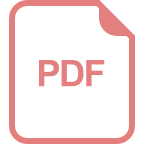
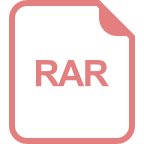
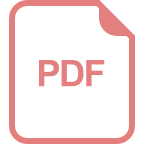
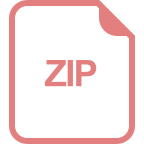
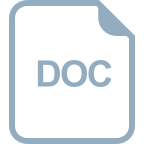







