用编程实现DIT-FFT算法;要求与DFT相比较,与系统自带FFT比较,图形化显示
时间: 2024-10-22 19:12:55 浏览: 48
DIT (Direct In-place Transform) 或称为 Decimation-in-Time 是快速傅立叶变换(Fast Fourier Transform,FFT)的一种常见变体,它通过逐次减少数据点的数量来进行计算,而不是一次性将所有数据移动到一端。
在Python中,你可以使用NumPy库来实现DIT FFT算法,例如Cooley-Tukey算法的变种。首先,你需要导入必要的模块:
```python
import numpy as np
```
然后,你可以编写一个函数来执行DIT FFT:
```python
def dit_fft(x):
N = x.shape[0]
if N <= 1:
return x
# Step 1: Divide the data into even and odd parts
X_even = x[::2]
X_odd = x[1::2]
# Step 2: Perform DIT on each part recursively
X_even = dit_fft(X_even)
X_odd = dit_fft(X_odd)
# Step 3: Combine the results using butterfly operations
result = np.zeros(N, dtype=x.dtype)
for k in range(int(N / 2)):
factor = np.exp(-2j * np.pi * k / N)
result[2 * k] = X_even[k] + factor * X_odd[k]
result[2 * k + 1] = X_even[k] - factor * X_odd[k]
return result
```
为了与系统自带的`numpy.fft.fft`函数比较性能并可视化结果,你可以创建两个版本的函数调用,并将它们的结果绘制出来:
```python
import matplotlib.pyplot as plt
# Generate a test signal
x = np.random.rand(1024)
# Your custom DIT FFT
custom_result = dit_fft(x)
# Compare with built-in FFT
builtin_result = np.fft.fft(x)
# Plot the magnitude of both results
plt.figure()
plt.plot(np.abs(custom_result), label="Custom DIT FFT")
plt.plot(np.abs(builtin_result), label="Built-in FFT")
plt.legend()
plt.xlabel("Frequency Index")
plt.ylabel("Magnitude")
plt.title("Comparison of Custom vs Built-in FFT")
# Show the graph
plt.show()
# Measure execution time
time_custom = %timeit -o dit_fft(x)
time_builtin = %timeit -o np.fft.fft(x)
print(f"Custom DIT FFT: {time_custom.best} seconds\nBuilt-in FFT: {time_builtin.best} seconds")
```
阅读全文
相关推荐
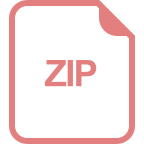
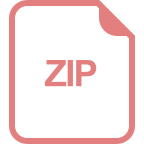
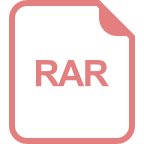

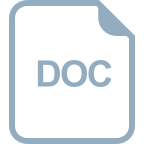

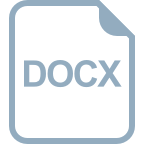
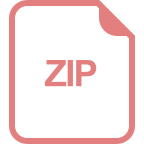
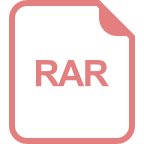
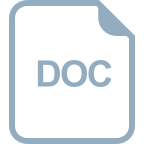
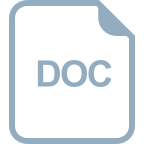
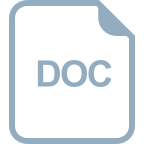
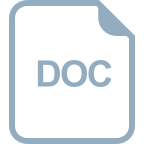
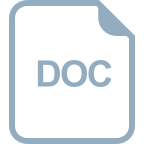
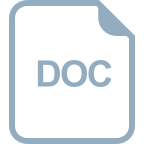
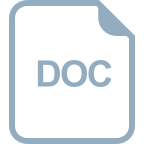
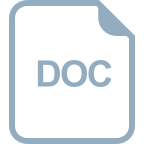

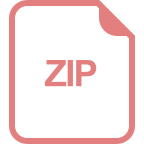