python获取cpu、内存、硬盘信息并发送给服务器
时间: 2024-10-16 09:19:37 浏览: 88
在Python中,你可以利用一些第三方库来获取CPU、内存和磁盘信息,然后通过网络发送到服务器。这里简单介绍一种常见的方式:
首先,你需要安装以下几个库:
1. psutil - 提供系统监控工具,如CPU、内存和磁盘信息。
2. socket - 用于创建网络连接和发送数据。
安装方法:
```bash
pip install psutil
```
接下来是一个简单的示例,展示如何获取信息并发送到服务器:
```python
import psutil
import socket
# 获取CPU信息
def get_cpu_info():
cpu_percent = psutil.cpu_percent(interval=1) # 每秒更新一次CPU使用率
cpu_count = psutil.cpu_count() # CPU核心数
return f"CPU信息:当前CPU使用率为{cpu_percent}%,总核心数为{cpu_count}"
# 获取内存信息
def get_memory_info():
memory = psutil.virtual_memory()
mem_usage = memory.percent
total = memory.total / (1024 ** 3) # MB转GB
return f"内存信息:内存使用率为{mem_usage}%,总内存为{total:.2f} GB"
# 获取磁盘信息(假设你想获取C盘)
def get_disk_info(path='C:\\'):
disk = psutil.disk_usage(path)
usage = disk.percent
total = disk.total / (1024 ** 3) # MB转GB
return f"C盘信息:磁盘使用率为{usage}%,总容量为{total:.2f} GB"
# 创建socket连接并发送数据
def send_data(host, port):
try:
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.connect((host, port))
cpu_data = get_cpu_info()
memory_data = get_memory_info()
disk_data = get_disk_info()
s.sendall(f"CPU信息:{cpu_data}\n内存信息:{memory_data}\n磁盘信息:{disk_data}".encode())
print("数据已发送")
except Exception as e:
print(f"发送失败:{e}")
# 发送到指定服务器
send_data("your_server_ip", 8080) # 将'your_server_ip'替换为实际服务器地址,端口可根据需要修改
阅读全文
相关推荐


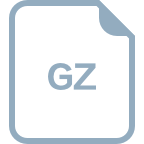













