JAVA或者js只传一个url 去下载文件
时间: 2024-09-11 18:04:13 浏览: 36
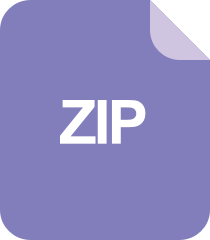
Java多个文件根据URL下载后打包zip导出.zip
在Java中,你可以使用`java.net.URL`和`java.io.InputStream`以及相关的I/O流来下载文件。下面是一个简单的示例:
```java
import java.io.FileOutputStream;
import java.io.InputStream;
import java.net.URL;
public class DownloadFile {
public static void downloadFile(String urlString) throws Exception {
URL url = new URL(urlString);
try (InputStream in = url.openStream();
FileOutputStream out = new FileOutputStream("file_name")) {
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = in.read(buffer)) != -1) {
out.write(buffer, 0, bytesRead);
}
}
}
public static void main(String[] args) {
String url = "http://example.com/file.zip";
try {
downloadFile(url);
System.out.println("Download completed.");
} catch (Exception e) {
System.out.println("Error occurred: " + e.getMessage());
}
}
}
```
对于JavaScript,通常会使用`fetch`或`XMLHttpRequest`配合`Blob`或`FileReader`来下载文件。以下是一个简单的`fetch`示例:
```javascript
async function downloadFile(url) {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const fileURL = window.URL.createObjectURL(new Blob([await response.blob()]));
const a = document.createElement('a');
a.href = fileURL;
a.download = 'file_name';
a.click();
window.URL.revokeObjectURL(fileURL); //释放资源
}
// 使用
downloadFile('http://example.com/file.zip');
```
阅读全文
相关推荐
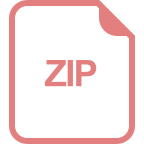
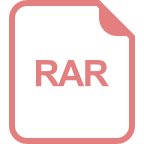
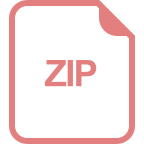
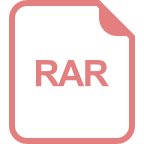
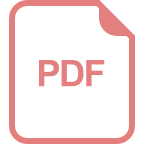
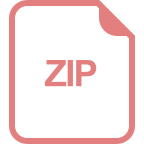
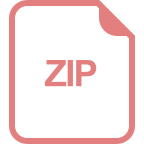
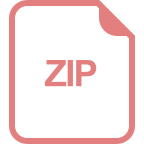
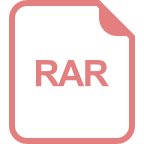
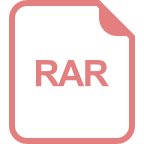
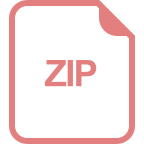
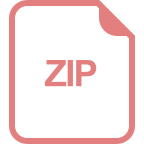
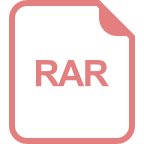
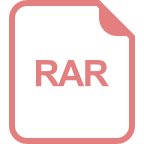
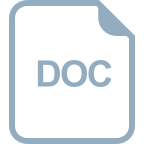
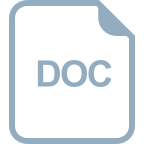
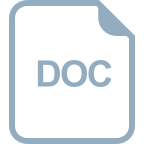
