如何在uniapp中实现子组件向父组件传值?
时间: 2024-10-11 21:13:36 浏览: 36
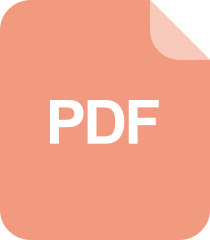
父组件中vuex方法更新state子组件不能及时更新并渲染的完美解决方法
在uniapp中,子组件向父组件传值可以通过props和emit机制来完成。这是与vue组件通信的基本方式:
**子组件向父组件传值(props)**[^2]:
在子组件中,你可以定义一个`props`属性并设置默认值,当子组件的状态改变时,这些值会自动传递到父组件。示例如下:
```html
<!-- 子组件 -->
<template>
<button @click="increaseValue">增加</button>
<text>{{ currentValue }}</text>
</template>
<script>
export default {
props: {
currentValue: {
type: Number,
default: 0
}
},
methods: {
increaseValue() {
this.currentValue++;
}
}
}
</script>
```
在父组件中接收并显示这个值:
```html
<!-- 父组件 -->
<template>
<child-component :currentValue="parentValue" />
<text>Parent Value: {{ parentValue }}</text>
</template>
<script>
import ChildComponent from '@/components/ChildComponent.vue'
export default {
components: {
ChildComponent
},
data() {
return {
parentValue: 0
}
}
}
</script>
```
**父组件触发事件向子组件传值(emit)**[^1]:
如果需要从父组件主动触发行为并传递数据给子组件,可以使用`$emit`方法。例如,在父组件点击按钮时更新子组件的数据:
```html
<!-- 父组件 -->
<template>
<button @click="passDataToChild">传递数据</button>
<child-component :value="receivedValue"></child-component>
</template>
<script>
export default {
methods: {
passDataToChild() {
this.receivedValue = 10;
// 或者触发自定义事件
this.$emit('customEvent', 10);
}
}
}
</script>
```
在子组件中监听这个事件:
```html
<!-- 子组件 -->
<template>
<text>Received Value: {{ receivedValue }}</text>
</template>
<script>
export default {
props: {
value: {
type: Number,
default: 0
}
},
methods: {
handleCustomEvent(value) {
this.value = value;
}
},
watch: {
'$root.$on': {
handler(event, arg) {
if (event === 'customEvent') {
this.handleCustomEvent(arg);
}
},
deep: true
}
}
}
</script>
```
这样,每当父组件调用`passDataToChild`或发送自定义事件时,子组件就会接收到新的值。
阅读全文
相关推荐
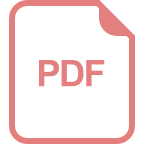
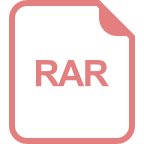















