uniapp父向子组件传值
时间: 2023-08-30 12:11:05 浏览: 116
在uni-app中,父组件向子组件传值可以通过props属性实现。
在父组件中,使用子组件时,可以通过子组件的属性props来传递数据。例如:
```html
<template>
<view>
<child :message="msg"></child>
</view>
</template>
<script>
import child from '@/components/child.vue'
export default {
components: {
child
},
data() {
return {
msg: 'Hello, child component!'
}
}
}
</script>
```
在子组件中,需要在props属性中声明接收父组件传递过来的数据。例如:
```html
<template>
<view>
<text>{{ message }}</text>
</view>
</template>
<script>
export default {
props: {
message: String
}
}
</script>
```
这样,父组件就可以向子组件传递数据,并在子组件中渲染出来了。
相关问题
uniapp父组件传值给子组件传值然后子组件显示
在UniApp中,父组件向子组件传递值通常通过props属性来实现。以下是步骤:
1. 父组件创建数据:
```javascript
<template>
<view>
<!-- 子组件 -->
<child-component :message="parentMessage" />
</view>
</template>
<script>
export default {
data() {
return {
parentMessage: "这是从父组件传递过来的值",
};
},
};
</script>
```
在这个例子中,`parentMessage`是一个数据属性,它会被作为`v-bind:`绑定到子组件`child-component`上。
2. 子组件接收并展示值:
```html
<!-- 子组件 child-component.vue -->
<template>
<text>{{ message }}</text>
</template>
<script>
import { watch } from '@dcloudio/uniview';
export default {
props: {
message: {
type: String,
required: true, // 如果不想让子组件为空,则设置required为true
},
},
created() {
watch("$route", () => {
// 如果需要根据路由动态更新消息,可以在这里处理
});
},
mounted() {
console.log('接收到的值:', this.message);
},
};
</script>
```
子组件会直接显示`message`属性的内容。
uniapp子组件向父组件传值
在uniapp中,子组件向父组件传值可以使用自定义事件。具体步骤如下:
1.在子组件中定义自定义事件
在子组件的 script 标签中,可以先定义一个方法,用于触发自定义事件并传递数据。如下所示:
```
methods: {
sendData() {
this.$emit('myevent', '这是要传递的数据')
}
}
```
其中 `this.$emit()` 方法用于触发自定义事件,第一个参数为自定义事件的名称,第二个参数为要传递的数据。
2.在父组件中监听自定义事件
在父组件中使用子组件时,可以在子组件上监听自定义事件,并在触发事件时执行相应的方法。如下所示:
```
<template>
<div>
<child-component @myevent="handleData"></child-component>
</div>
</template>
<script>
import childComponent from '@/components/child-component'
export default {
components: {
childComponent
},
methods: {
handleData(data) {
console.log(data) // 输出:这是要传递的数据
}
}
}
</script>
```
在子组件上使用 `@myevent` 监听自定义事件,并在触发事件时执行 `handleData` 方法。在该方法中可以获取到子组件传递过来的数据。
需要注意的是,父组件中监听的自定义事件名称应该与子组件中触发的自定义事件名称一致,否则无法传递数据。
阅读全文
相关推荐
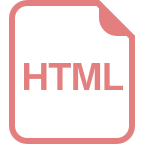
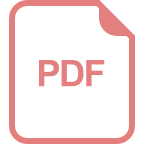













