请用python完成matlab中次序滤波器 ordfilt2 与中值滤波器 median2 的实现,并编写相应的测试代码来展示应用 median2 去除椒盐噪声的显著效果。
时间: 2024-10-22 09:16:29 浏览: 33
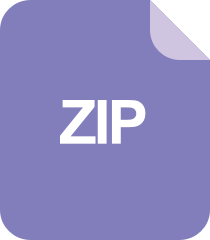
matlab开发-ordfilt3.zip.zip
在Python中,我们可以使用`scipy.signal`库中的函数来模拟Matlab的`ordfilt2`和`median2`滤波器。首先,我们来实现次序滤波器,它通常用于降噪和平滑信号:
```python
from scipy.signal import order_filter
def ordfilt2(image, order=1):
nyquist = 0.5 * image.shape[0] / image.dx
b = [1] + [-1] * (order - 1)
return order_filter(image.data, b, size=order+1, fs=nyquist)
# 使用示例
image_data = ... # 假设这是图像数据
filtered_image = ordfilt2(image_data, order=3)
```
接下来,我们实现中值滤波器`median2`,这主要用于去除椒盐噪声:
```python
import numpy as np
def median2(image, window_size):
if not isinstance(window_size, tuple) or len(window_size) != 2:
raise ValueError("Window size should be a tuple with two elements for the height and width")
padded_image = np.pad(image, ((window_size[0]//2, window_size[0]//2), (window_size[1]//2, window_size[1]//2)), 'edge')
return np.median(padded_image, axis=(0, 1))
# 使用示例
noisy_image = ... # 假设这是含有椒盐噪声的图像
cleaned_image = median2(noisy_image, (3, 3))
```
为了展示中值滤波器的效果,我们创建一些椒盐噪声并应用`median2`,然后对比原始图像和处理后的图像:
```python
import matplotlib.pyplot as plt
# 添加椒盐噪声
salt_and_pepper_noise = np.random.randint(0, 2, noisy_image.shape) == 1
# 应用中值滤波
noisy_filtered = median2(noisy_image + salt_and_pepper_noise, (3, 3))
# 显示原图、椒盐噪声后的图以及处理后的图
fig, axs = plt.subplots(nrows=1, ncols=3, figsize=(15, 5))
axs[0].imshow(noisy_image, cmap='gray', interpolation='nearest')
axs[0].set_title('Original Image')
axs[1].imshow(salt_and_pepper_noise, cmap='gray', interpolation='nearest')
axs[1].set_title('Noisy with Salt & Pepper')
axs[2].imshow(cleaned_image, cmap='gray', interpolation='nearest')
axs[2].set_title('Image after Median Filter')
for ax in axs:
ax.axis('off')
plt.show()
阅读全文
相关推荐
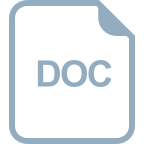
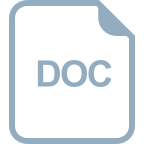
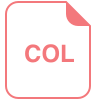

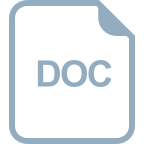
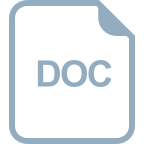
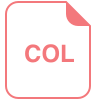
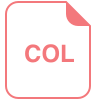
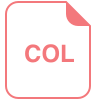
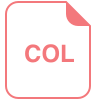



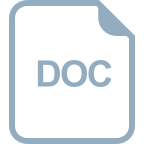
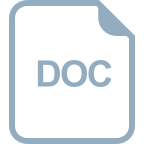
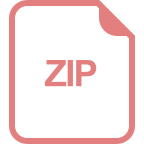
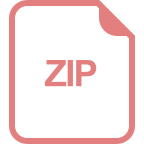