vue2+js + antd,在一个遍历的下拉框中,所有选择框(选择框是多选的)共用的一个选项列表数据,如何确保 之前选过的选项,在其他选择框之前选过的禁用呢?代码
时间: 2024-12-23 20:25:10 浏览: 6
在Vue 2中使用Ant Design组件,并且处理复选框选择项的禁用状态,可以借助Vuex或自定义的state管理来存储已经选择的选项,然后在渲染下拉框时动态更新其选项的状态。以下是基本步骤:
1. 创建Vuex store 或者在单页面应用中定义一个局部状态来存储已选择的选项:
```javascript
// 使用Vuex
import { createStore } from 'vuex';
const store = createStore({
state: {
selectedOptions: []
},
mutations: {
setSelectedOption(state, option) {
if (!state.selectedOptions.includes(option)) {
state.selectedOptions.push(option);
}
},
removeSelectedOption(state, option) {
state.selectedOptions = state.selectedOptions.filter(item => item !== option);
}
}
});
// 或者在单页组件内部定义
data() {
return {
selectedOptions: []
};
}
methods: {
selectOption(option) {
this.selectedOptions.push(option);
},
deselectOption(option) {
const index = this.selectedOptions.findIndex(item => item === option);
if (index > -1) {
this.selectedOptions.splice(index, 1);
}
}
}
```
2. 当你在遍历的下拉框中渲染选项时,检查当前选项是否已经在已选列表里:
```vue
<template>
<div>
<c-select v-for="(option, index) in options" :key="index" :disabled="option.disabled || isSelected(option.value)" @on-change="onChange">
<!-- ... -->
</c-select>
</div>
</template>
<script>
export default {
props: ['options'],
computed: {
isSelected(optionValue) {
return this.store.state.selectedOptions.includes(optionValue); // 使用Vuex
// 或者 this.selectedOptions.includes(optionValue); // 使用单页面组件内的状态
},
},
methods: {
onChange(value) {
this.$store.commit('setSelectedOption', value); // 使用Vuex
// 或者 this.selectedOptions.includes(value) ? this.deselectOption(value) : this.selectOption(value); // 使用单页面组件内的状态
}
}
};
</script>
```
以上代码中,我们通过计算属性 `isSelected` 检查选项是否已被选中,并基于这个判断调整下拉框的 `disabled` 属性。当用户改变选项时,我们相应地更新状态。
阅读全文
相关推荐
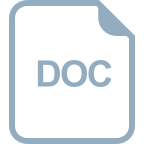
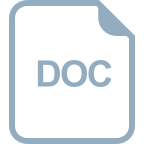
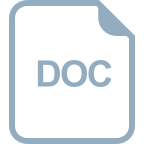



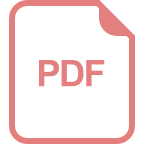
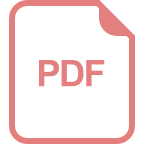
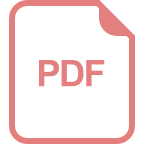
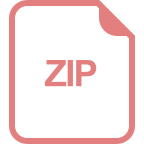
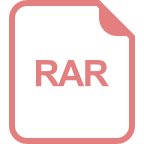
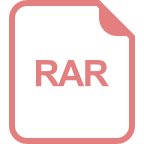
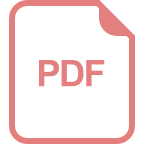
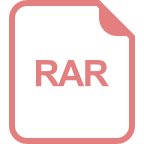
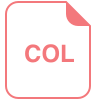
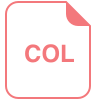


