C语言自定义函数实现四则运算
时间: 2024-04-14 07:21:32 浏览: 21
C语言自定义函数实现四则运算的方法是通过将中缀表达式转换为后缀表达式,然后利用栈来实现计算。具体步骤如下:
1. 定义一个栈,用于存储操作数和运算符。
2. 将中缀表达式转换为后缀表达式,即将运算符按照优先级顺序加入到栈中,操作数直接输出。
3. 遍历后缀表达式,如果是操作数,则将其压入栈中;如果是运算符,则弹出栈顶的两个操作数进行计算,并将结果压入栈中。
4. 最后栈中只剩下一个元素,即为计算结果。
下面是一个简单的实现示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_STACK_SIZE 100
double stack[MAX_STACK_SIZE];
int top = -1;
void push(double value) {
if (top >= MAX_STACK_SIZE - 1) {
printf("Stack overflow\n");
exit(1);
}
stack[++top] = value;
}
double pop() {
if (top < 0) {
printf("Stack underflow\n");
exit(1);
}
return stack[top--];
}
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/' || c == '%';
}
int precedence(char c) {
if (c == '+' || c == '-') {
return 1;
} else if (c == '*' || c == '/' || c == '%') {
return 2;
} else {
return 0;
}
}
void infix_to_postfix(char infix[], char postfix[]) {
int i, j;
char c;
for (i = j = 0; infix[i] != '\0'; i++) {
if (isdigit(infix[i])) {
postfix[j++] = infix[i];
} else if (is_operator(infix[i])) {
while (top >= 0 && precedence(stack[top]) >= precedence(infix[i])) {
postfix[j++] = pop();
}
push(infix[i]);
} else if (infix[i] == '(') {
push(infix[i]);
} else if (infix[i] == ')') {
while ((c = pop()) != '(') {
postfix[j++] = c;
}
}
}
while (top >= 0) {
postfix[j++] = pop();
}
postfix[j] = '\0';
}
double evaluate_postfix(char postfix[]) {
int i;
double op1, op2;
for (i = 0; postfix[i] != '\0'; i++) {
if (isdigit(postfix[i])) {
push(postfix[i] - '0');
} else if (is_operator(postfix[i])) {
op2 = pop();
op1 = pop();
switch (postfix[i]) {
case '+':
push(op1 + op2);
break;
case '-':
push(op1 - op2);
break;
case '*':
push(op1 * op2);
break;
case '/':
push(op1 / op2);
break;
case '%':
push((int)op1 % (int)op2);
break;
}
}
}
return pop();
}
int main() {
char infix[100], postfix[100];
double result;
printf("Enter an infix expression: ");
scanf("%s", infix);
infix_to_postfix(infix, postfix);
printf("Postfix expression: %s\n", postfix);
result = evaluate_postfix(postfix);
printf("Result: %.2lf\n", result);
return 0;
}
```
相关推荐
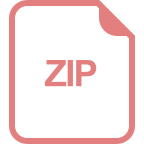










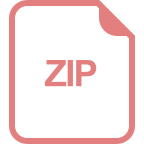