C语言实现动态内存学生管理系统
时间: 2024-11-08 13:13:02 浏览: 6
C语言实现动态内存的学生管理系统通常涉及以下几个步骤:
1. **结构体定义**:首先,创建一个结构体,用于表示学生的信息,如学号、姓名、成绩等。
```c
typedef struct {
int id;
char name[50];
float score;
} Student;
```
2. **动态内存分配**:使用`malloc()`函数为每个新添加的学生分配内存。
```c
Student* create_student(int id, const char* name, float score) {
Student* new_student = (Student*) malloc(sizeof(Student));
if (new_student != NULL) {
new_student->id = id;
strcpy(new_student->name, name);
new_student->score = score;
} else {
printf("Memory allocation failed.\n");
return NULL;
}
return new_student;
}
```
3. **管理操作**:定义一些函数来添加、删除和更新学生信息,同时要考虑释放内存以避免内存泄漏。
```c
void add_student(Student** students, int* count, ...) {
va_list args;
va_start(args, count);
Student* new_student = create_student(va_arg(args, int), va_arg(args, char*), va_arg(args, float));
if (new_student != NULL) {
students[*count] = new_student;
(*count)++;
}
va_end(args);
}
void remove_student(Student** students, int index) {
free(students[index]);
memmove(students + index, students + index + 1, sizeof(Student) * ((*count) - index - 1));
(*count)--;
}
// 更改分数示例
void update_score(Student* student, float new_score) {
student->score = new_score;
}
```
4. **主程序和数据结构**:在主程序中,可以初始化一个动态数组来存储学生,并调用上述函数进行操作。
```c
int main() {
int num_students = 0;
Student* students = malloc(sizeof(Student));
// 使用系统管理功能...
for (Student* s : students) {
free(s);
}
free(students);
return 0;
}
```
阅读全文
相关推荐
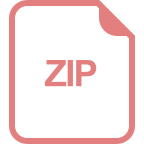
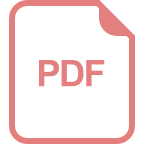
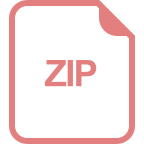
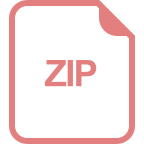
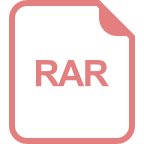
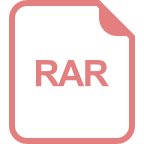
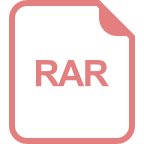
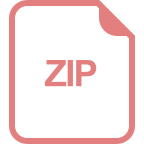
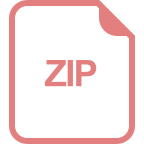
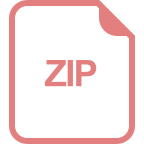
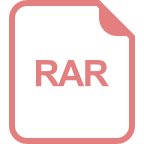
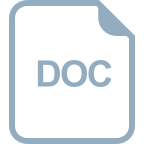
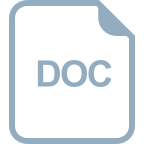
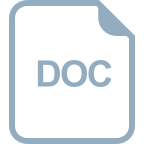
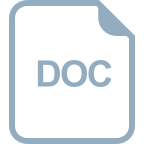
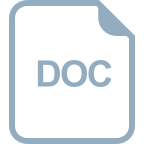
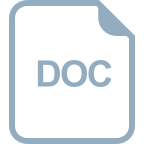
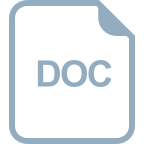
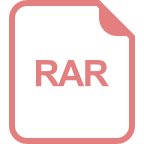