android studio贷款计算器
时间: 2023-11-02 16:07:55 浏览: 228
安卓贷款计算器源码是一个使用Android Studio编写的应用程序,它支持四种贷款类型计算,包括等额本金、等额本息、反推等额本金年利息和反推等额本息年利息。此外,还有一个简单的房贷计算器,它使用了一些新颖的技术,如CheckBox图标的改变、EditText格式的改变、Spinner的使用和房贷的计算逻辑。如果你想获取这个应用程序的源代码,可以访问上面提供的链接。
相关问题
帮我用Android Studio 写一个贷款计算器的程序
以下是一个简单的房贷计算器的Android Studio程序,其中包括CheckBox图标的改变、EditText格式的改变、Spinner的使用和房贷的计算逻辑。你可以根据自己的需要进行修改和扩展。
```java
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.text.Editable;
import android.text.TextWatcher;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.Spinner;
import android.widget.TextView;
import java.text.DecimalFormat;
public class MainActivity extends AppCompatActivity implements AdapterView.OnItemSelectedListener {
private EditText etLoanAmount, etLoanTerm, etInterestRate;
private TextView tvMonthlyPayment, tvTotalPayment, tvTotalInterest;
private CheckBox cbTaxes, cbInsurance;
private Spinner spLoanType;
private Button btnCalculate;
private double loanAmount, interestRate, monthlyPayment, totalPayment, totalInterest;
private int loanTerm, loanType;
private boolean taxes, insurance;
private DecimalFormat df = new DecimalFormat("#.##");
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
etLoanAmount = findViewById(R.id.et_loan_amount);
etLoanTerm = findViewById(R.id.et_loan_term);
etInterestRate = findViewById(R.id.et_interest_rate);
tvMonthlyPayment = findViewById(R.id.tv_monthly_payment);
tvTotalPayment = findViewById(R.id.tv_total_payment);
tvTotalInterest = findViewById(R.id.tv_total_interest);
cbTaxes = findViewById(R.id.cb_taxes);
cbInsurance = findViewById(R.id.cb_insurance);
spLoanType = findViewById(R.id.sp_loan_type);
btnCalculate = findViewById(R.id.btn_calculate);
ArrayAdapter<CharSequence> adapter = ArrayAdapter.createFromResource(this,
R.array.loan_types, android.R.layout.simple_spinner_item);
adapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
spLoanType.setAdapter(adapter);
spLoanType.setOnItemSelectedListener(this);
etLoanAmount.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
if (!s.toString().equals("")) {
loanAmount = Double.parseDouble(s.toString());
} else {
loanAmount = 0;
}
}
@Override
public void afterTextChanged(Editable s) {}
});
etLoanTerm.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
if (!s.toString().equals("")) {
loanTerm = Integer.parseInt(s.toString());
} else {
loanTerm = 0;
}
}
@Override
public void afterTextChanged(Editable s) {}
});
etInterestRate.addTextChangedListener(new TextWatcher() {
@Override
public void beforeTextChanged(CharSequence s, int start, int count, int after) {}
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
if (!s.toString().equals("")) {
interestRate = Double.parseDouble(s.toString());
} else {
interestRate = 0;
}
}
@Override
public void afterTextChanged(Editable s) {}
});
cbTaxes.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
taxes = cbTaxes.isChecked();
}
});
cbInsurance.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
insurance = cbInsurance.isChecked();
}
});
btnCalculate.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
switch (loanType) {
case 0:
calculateEqualPrincipal();
break;
case 1:
calculateEqualInstallment();
break;
case 2:
calculateReverseEqualPrincipal();
break;
case 3:
calculateReverseEqualInstallment();
break;
}
tvMonthlyPayment.setText(df.format(monthlyPayment));
tvTotalPayment.setText(df.format(totalPayment));
tvTotalInterest.setText(df.format(totalInterest));
}
});
}
private void calculateEqualPrincipal() {
double monthlyInterestRate = interestRate / 12;
double principal = loanAmount / loanTerm;
double interest = loanAmount * monthlyInterestRate;
monthlyPayment = principal + interest;
totalPayment = monthlyPayment * loanTerm;
totalInterest = totalPayment - loanAmount;
if (taxes) {
totalPayment += loanTerm * 100;
}
if (insurance) {
totalPayment += loanTerm * 50;
}
}
private void calculateEqualInstallment() {
double monthlyInterestRate = interestRate / 12;
double monthlyPaymentRate = monthlyInterestRate * Math.pow(1 + monthlyInterestRate, loanTerm)
/ (Math.pow(1 + monthlyInterestRate, loanTerm) - 1);
monthlyPayment = loanAmount * monthlyPaymentRate;
totalPayment = monthlyPayment * loanTerm;
totalInterest = totalPayment - loanAmount;
if (taxes) {
totalPayment += loanTerm * 100;
}
if (insurance) {
totalPayment += loanTerm * 50;
}
}
private void calculateReverseEqualPrincipal() {
double monthlyInterestRate = interestRate / 12;
double principal = loanAmount / loanTerm;
double interest = loanAmount * monthlyInterestRate;
double monthlyPayment1 = principal + interest;
double monthlyPayment2 = monthlyPayment1 + principal * monthlyInterestRate;
double monthlyPayment3 = monthlyPayment2 + principal * monthlyInterestRate;
double monthlyPayment4 = monthlyPayment3 + principal * monthlyInterestRate;
double monthlyPayment5 = monthlyPayment4 + principal * monthlyInterestRate;
double monthlyPayment6 = monthlyPayment5 + principal * monthlyInterestRate;
double monthlyPayment7 = monthlyPayment6 + principal * monthlyInterestRate;
double monthlyPayment8 = monthlyPayment7 + principal * monthlyInterestRate;
double monthlyPayment9 = monthlyPayment8 + principal * monthlyInterestRate;
double monthlyPayment10 = monthlyPayment9 + principal * monthlyInterestRate;
double monthlyPayment11 = monthlyPayment10 + principal * monthlyInterestRate;
double monthlyPayment12 = monthlyPayment11 + principal * monthlyInterestRate;
monthlyPayment = (monthlyPayment1 + monthlyPayment2 + monthlyPayment3 + monthlyPayment4
+ monthlyPayment5 + monthlyPayment6 + monthlyPayment7 + monthlyPayment8
+ monthlyPayment9 + monthlyPayment10 + monthlyPayment11 + monthlyPayment12) / 12;
totalPayment = monthlyPayment * loanTerm;
totalInterest = totalPayment - loanAmount;
if (taxes) {
totalPayment += loanTerm * 100;
}
if (insurance) {
totalPayment += loanTerm * 50;
}
}
private void calculateReverseEqualInstallment() {
double monthlyInterestRate = interestRate / 12;
double monthlyPaymentRate = monthlyInterestRate * Math.pow(1 + monthlyInterestRate, loanTerm)
/ (Math.pow(1 + monthlyInterestRate, loanTerm) - 1);
double monthlyPayment1 = loanAmount * monthlyPaymentRate;
double monthlyPayment2 = (loanAmount - monthlyPayment1) * monthlyInterestRate + monthlyPayment1;
double monthlyPayment3 = (loanAmount - monthlyPayment1 - monthlyPayment2) * monthlyInterestRate + monthlyPayment2;
double monthlyPayment4 = (loanAmount - monthlyPayment1 - monthlyPayment2 - monthlyPayment3) * monthlyInterestRate + monthlyPayment3;
double monthlyPayment5 = (loanAmount - monthlyPayment1 - monthlyPayment2 - monthlyPayment3 - monthlyPayment4) * monthlyInterestRate + monthlyPayment4;
double monthlyPayment6 = (loanAmount - monthlyPayment1 - monthlyPayment2 - monthlyPayment3 - monthlyPayment4 - monthlyPayment5) * monthlyInterestRate + monthlyPayment5;
double monthlyPayment7 = (loanAmount - monthlyPayment1 - monthlyPayment2 - monthlyPayment3 - monthlyPayment4 - monthlyPayment5 - monthlyPayment6) * monthlyInterestRate + monthlyPayment6;
double monthlyPayment8 = (loanAmount - monthlyPayment1 - monthlyPayment2 - monthlyPayment3 - monthlyPayment4 - monthlyPayment5 - monthlyPayment6 - monthlyPayment7) * monthlyInterestRate + monthlyPayment7;
double monthlyPayment9 = (loanAmount - monthlyPayment1 - monthlyPayment2 - monthlyPayment3 - monthlyPayment4 - monthlyPayment5 - monthlyPayment6 - monthlyPayment7 - monthlyPayment8) * monthlyInterestRate + monthlyPayment8;
double monthlyPayment10 = (loanAmount - monthlyPayment1 - monthlyPayment2 - monthlyPayment3 - monthlyPayment4 - monthlyPayment5 - monthlyPayment6 - monthlyPayment7 - monthlyPayment8 - monthlyPayment9) * monthlyInterestRate + monthlyPayment9;
double monthlyPayment11 = (loanAmount - monthlyPayment1 - monthlyPayment2 - monthlyPayment3 - monthlyPayment4 - monthlyPayment5 - monthlyPayment6 - monthlyPayment7 - monthlyPayment8 - monthlyPayment9 - monthlyPayment10) * monthlyInterestRate + monthlyPayment10;
double monthlyPayment12 = (loanAmount - monthlyPayment1 - monthlyPayment2 - monthlyPayment3 - monthlyPayment4 - monthlyPayment5 - monthlyPayment6 - monthlyPayment7 - monthlyPayment8 - monthlyPayment9 - monthlyPayment10 - monthlyPayment11) * monthlyInterestRate + monthlyPayment11;
monthlyPayment = (monthlyPayment1 + monthlyPayment2 + monthlyPayment3 + monthlyPayment4
+ monthlyPayment5 + monthlyPayment6 + monthlyPayment7 + monthlyPayment8
+ monthlyPayment9 + monthlyPayment10 + monthlyPayment11 + monthlyPayment12) / 12;
totalPayment = monthlyPayment * loanTerm;
totalInterest = totalPayment - loanAmount;
if (taxes) {
totalPayment += loanTerm * 100;
}
if (insurance) {
totalPayment += loanTerm * 50;
}
}
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
loanType = position;
}
@Override
public void onNothingSelected(AdapterView<?> parent) {}
}
```
阅读全文
相关推荐
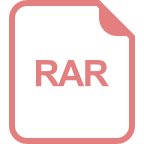
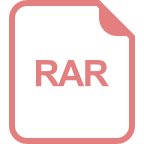
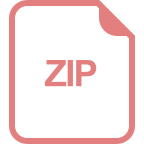
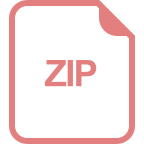
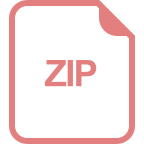
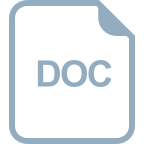
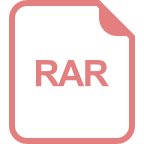
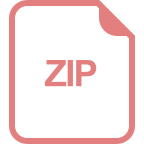
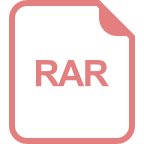
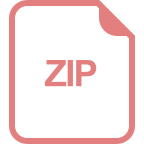
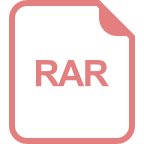
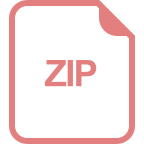
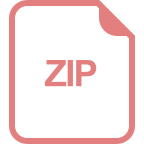
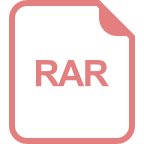
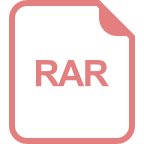
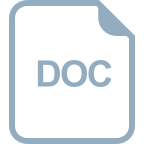