c#如何模拟鼠标单击
时间: 2023-12-14 15:34:43 浏览: 109
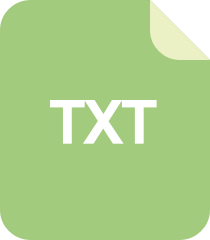
c#中用api控制鼠标

以下是C#模拟鼠标单击的示例代码:
```csharp
using System;
using System.Runtime.InteropServices;
public class MouseSimulator
{
[DllImport("user32.dll", SetLastError = true)]
static extern uint SendInput(uint nInputs, INPUT[] pInputs, int cbSize);
[StructLayout(LayoutKind.Sequential)]
struct INPUT
{
public uint type;
public MOUSEINPUT mi;
}
[StructLayout(LayoutKind.Sequential)]
struct MOUSEINPUT
{
public int dx;
public int dy;
public uint mouseData;
public uint dwFlags;
public uint time;
public IntPtr dwExtraInfo;
}
const uint MOUSEEVENTF_LEFTDOWN = 0x0002;
const uint MOUSEEVENTF_LEFTUP = 0x0004;
public static void SimulateLeftClick(int x, int y)
{
INPUT[] inputs = new INPUT[2];
inputs[0].type = 0; // INPUT_MOUSE
inputs[0].mi.dx = x;
inputs[0].mi.dy = y;
inputs[0].mi.mouseData = 0;
inputs[0].mi.dwFlags = MOUSEEVENTF_LEFTDOWN;
inputs[0].mi.time = 0;
inputs[0].mi.dwExtraInfo = IntPtr.Zero;
inputs[1].type = 0; // INPUT_MOUSE
inputs[1].mi.dx = x;
inputs[1].mi.dy = y;
inputs[1].mi.mouseData = 0;
inputs[1].mi.dwFlags = MOUSEEVENTF_LEFTUP;
inputs[1].mi.time = 0;
inputs[1].mi.dwExtraInfo = IntPtr.Zero;
SendInput(2, inputs, Marshal.SizeOf(typeof(INPUT)));
}
}
```
上述代码中,我们使用了Windows API中的SendInput函数来模拟鼠标单击事件。具体来说,我们定义了一个MOUSEINPUT结构体来描述鼠标事件的各个参数,然后将其封装在一个INPUT结构体中,最后调用SendInput函数来发送模拟的鼠标事件。
要模拟鼠标单击,我们需要调用两次SendInput函数,第一次发送鼠标按下事件,第二次发送鼠标释放事件。在这个示例代码中,我们将这两个事件封装在了SimulateLeftClick函数中,该函数接受鼠标单击的坐标作为参数。
阅读全文
相关推荐
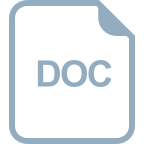
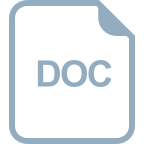
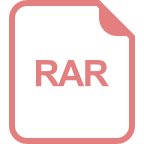

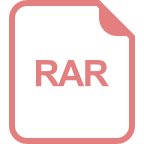
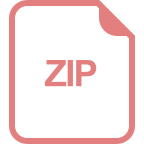
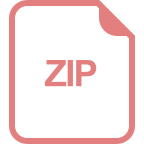
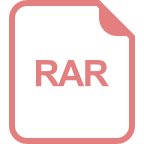
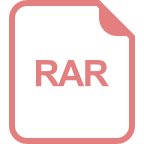
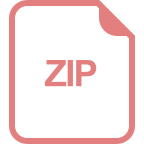
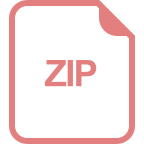
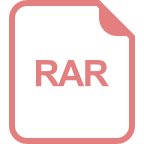
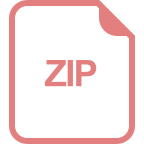
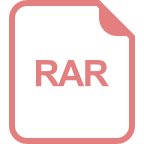
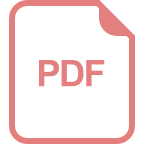
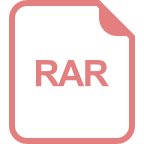
