编写一个实验程序,假设串用string对象表示,给定两个字符串 s和t,求串t在串s中不重叠出现的次数,如果不是子串,返回0。例如,s="aaaab",t="aa",则t在s中出现两次,用相关数据进行测试,用c++语言编写
时间: 2024-12-06 14:21:44 浏览: 14
为了编写这个实验程序,我们可以使用C++中的`std::string`类以及循环来遍历并检查字符串。下面是一个简单的示例代码,它会找到字符串`t`在字符串`s`中非重叠出现的次数:
```cpp
#include <iostream>
#include <string>
int countNonOverlappingOccurrences(const std::string& s, const std::string& t) {
int count = 0;
size_t pos = 0;
while ((pos = s.find(t, pos)) != std::string::npos) {
// 如果当前位置等于上一次查找的位置加上`t`的长度,则说明有重叠,跳过计数
if (pos == 0 || pos + t.length() > s.length()) {
pos += t.length();
} else {
++count;
pos += t.length(); // 移动到下一个可能的位置
}
}
return count;
}
int main() {
std::string s = "aaaab";
std::string t = "aa";
int occurrences = countNonOverlappingOccurrences(s, t);
std::cout << "String 't' appears " << occurrences << " times in 's' without overlap.\n";
// 测试其他数据
std::string test_s1 = "abcabcabc";
std::string test_t1 = "abc";
std::cout << "In test_s1 ('" << test_s1 << "'), " << countNonOverlappingOccurrences(test_s1, test_t1) << " times.\n";
std::string test_s2 = "hello";
std::string test_t2 = "ll";
std::cout << "In test_s2 ('" << test_s2 << "'), " << countNonOverlappingOccurrences(test_s2, test_t2) << " times.\n";
return 0;
}
```
在这个程序中,我们首先定义了一个名为`countNonOverlappingOccurrences`的函数,用于计算`t`在`s`中的非重叠出现次数。然后在`main`函数中,我们进行了几个例子的测试。
阅读全文
相关推荐
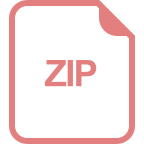
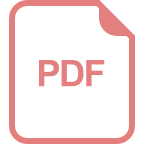


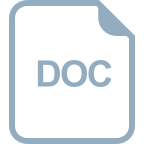
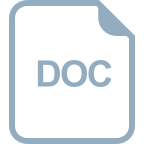












