用Python写一个高级浪漫动态粒子跳动爱心代码
时间: 2024-11-13 13:20:57 浏览: 86
在Python中,我们可以使用`pygame`库来创建一个简单的动画效果,模拟爱心动态粒子跳动。下面是一个简化的示例代码,它在一个窗口中生成随机移动的心形粒子:
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置窗口大小和标题
win_width, win_height = 600, 400
window = pygame.display.set_mode((win_width, win_height))
pygame.display.set_caption("动态爱心粒子")
# 粒子颜色和心形模板
particle_color = (255, 0, 0)
heart_template = pygame.Surface((50, 50), flags=pygame.SRCALPHA)
# 定义心形形状
def draw_heart(heart_template):
# 画出心形,你可以根据需要调整这个函数
# 这里只是一个简化示例
pygame.draw.polygon(heart_template, particle_color, [
(0, 50),
(50, 0),
(100, 50),
(75, 100),
(25, 100)
])
# 主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 清空屏幕
window.fill((0, 0, 0))
# 创建新的粒子
num_particles = random.randint(10, 50)
particles = [pygame.Rect(random.randint(0, win_width - heart_template.get_width()),
random.randint(0, win_height - heart_template.get_height()),
heart_template.get_width(), heart_template.get_height())
for _ in range(num_particles)]
# 更新和绘制粒子
for particle in particles:
draw_heart(heart_template)
particle.center += (random.uniform(-2, 2), random.uniform(-2, 2)) # 随机移动
if not (0 <= particle.x < win_width and 0 <= particle.y < win_height): # 如果粒子超出边界
particle.x, particle.y = random.randint(0, win_width - heart_template.get_width()),
random.randint(0, win_height - heart_template.get_height())
# 绘制到窗口
window.blit(heart_template, particles)
# 更新显示
pygame.display.update()
# 退出游戏
pygame.quit()
```
运行此代码会打开一个新的窗口,显示一系列红色爱心粒子随机跳跃。注意这只是一个基础版本,你还可以添加更复杂的视觉效果、音乐背景或者其他交互。
阅读全文
相关推荐
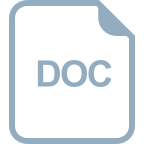
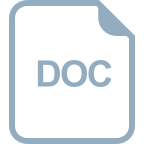
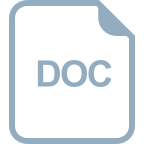











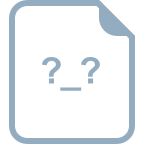
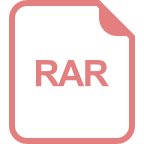
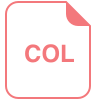
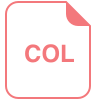
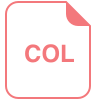