用BF算法解决串匹配问题,需要用C语言代码写出
时间: 2024-12-11 13:35:37 浏览: 16
Bloom Filter是一种空间效率极高的概率型数据结构,常用于快速判断一个元素是否在一个集合中。然而,它并不是用来解决精确的串匹配问题的(如KMP、ACM等),而是用于高效地排除不在集合的可能性。
如果你想要解决精确的字符串匹配问题,比如在文本中查找特定模式(如正则表达式),通常会使用更为专业的算法,例如Knuth-Morris-Pratt(KMP)、Boyer-Moore等。
然而,如果你想了解如何使用C语言实现一种简单的字符串搜索算法,如顺序查找(即从头到尾逐字符对比),可以参考下面这个简单的示例:
```c
#include <stdio.h>
#include <string.h>
// 检查目标字符串是否包含主字符串
int simple_string_search(const char* target, const char* pattern) {
int i = 0, j = 0;
while (i < strlen(target)) {
if (target[i] == pattern[j]) {
i++;
j++;
} else {
// 如果目标字符不等于模式字符,直接跳过模式长度尝试再次比较
if (j != 0) {
j = 0; // 回退模式指针
} else {
i++; // 继续检查下一个目标字符
}
}
// 如果找到整个模式,返回模式开始位置
if (j == strlen(pattern)) {
return i - j;
}
}
return -1; // 主字符串未找到
}
int main() {
const char* text = "Hello, world!";
const char* pattern = "world";
int result = simple_string_search(text, pattern);
if (result != -1) {
printf("Pattern found at position %d\n", result);
} else {
printf("Pattern not found in the text.\n");
}
return 0;
}
```
阅读全文
相关推荐




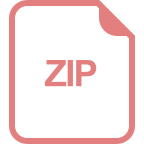













