用C语言简单写出串匹配的BF算法,并给出具体实例,要求解析
时间: 2024-10-22 14:19:55 浏览: 11
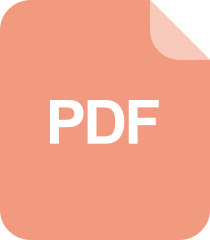
c语言中使用BF-KMP算法实例
在C语言中,Boyer-Moore (BM) 算法是一种常用的字符串搜索算法,它通过预处理模式串,避免了不必要的字符比较,提高了查找效率。下面是一个简单的 Boyer-Moore 搜索算法的实现:
```c
#include <stdio.h>
#include <string.h>
#define MAX haystack_len + needle_len
int bad_char[] = {0}; // 初始化数组存储模式串中每个字符最后一次出现的位置
int good_suff[]; // 初始化数组存储模式串的后缀跳过值
void preprocess(char* needle) {
int i;
for (i = needle_len - 2; i >= 0; i--) {
bad_char[needle[i]] = i + 1;
}
good_suff[needle[needle_len - 1]] = needle_len - 1; // 最后一个字符直接跳过自身
for (i = needle_len - 2; i >= 0; i--) {
if (needle[i] != needle[i + 1]) {
good_suff[needle[i]] = i + 1;
} else {
good_suff[needle[i]] = good_suff[needle[i + 1]];
}
}
}
int BM_search(char* haystack, char* needle) {
preprocess(needle);
int h_pos = 0, n_pos = 0;
while (h_pos < strlen(haystack)) {
if (haystack[h_pos] == needle[n_pos]) {
if (n_pos == needle_len - 1) {
printf("Pattern found at position %d in the haystack\n", h_pos - n_pos + 1);
return h_pos;
}
h_pos++, n_pos++;
} else {
int skip_distance = bad_char[haystack[h_pos]] > n_pos - good_suff[needle[n_pos]] ? bad_char[haystack[h_pos]] : n_pos - good_suff[needle[n_pos]];
h_pos += skip_distance;
n_pos = 0;
}
}
return -1; // 如果找不到,则返回 -1
}
int main() {
char haystack[] = "ABABCABABCD";
char needle[] = "ABC";
BM_search(haystack, needle);
return 0;
}
```
在这个例子中,`preprocess()` 函数用于初始化 `bad_char` 和 `good_suff` 数组。`BM_search()` 函数则是实际的搜索过程,通过 `h_pos` 和 `n_pos` 分别跟踪 Haystack 和 Needle 的当前位置。当字符相等时,同时移动;否则,根据 BM 算法计算跳过的距离并移动。
阅读全文
相关推荐
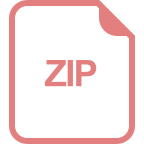
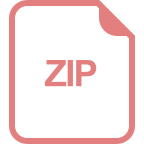
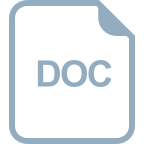
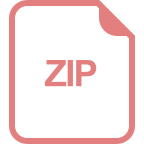
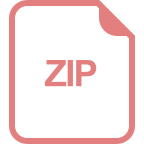
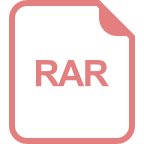
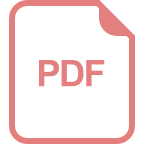
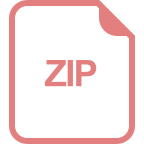
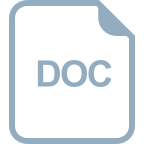
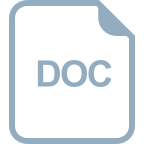
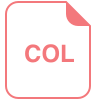
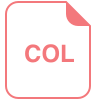
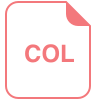
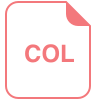
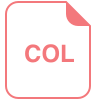