python实现一个二维空间点对象的 Poin2D 类, 要求该类包含用于返回点对象的位 置、到其他点之间距离、支持点移动、判断两点之间是否相等的基本功能; 在 Point2D 类的基础上,采用继承方式定义三维空间 Point3D 类, 并实现 Point2D 类中所有内置功能函数。
时间: 2024-04-14 18:29:08 浏览: 18
下面是一个示例的 Point2D 类的实现:
```python
import math
class Point2D:
def __init__(self, x, y):
self.x = x
self.y = y
def get_position(self):
return (self.x, self.y)
def distance_to(self, other):
dx = self.x - other.x
dy = self.y - other.y
return math.sqrt(dx**2 + dy**2)
def move(self, dx, dy):
self.x += dx
self.y += dy
def __eq__(self, other):
return self.x == other.x and self.y == other.y
```
然后,我们可以使用继承来实现 Point3D 类:
```python
class Point3D(Point2D):
def __init__(self, x, y, z):
super().__init__(x, y)
self.z = z
def get_position(self):
return (self.x, self.y, self.z)
```
这样,Point3D 类就继承了 Point2D 类中的所有基本功能函数,并且还添加了一个新的维度 z。可以通过调用 `get_position` 方法来获取点的位置信息,包括 x、y 和 z 坐标。
相关推荐
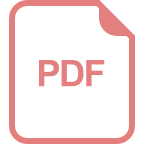
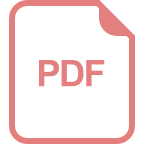
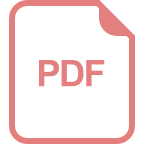













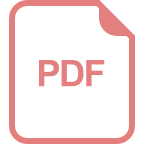
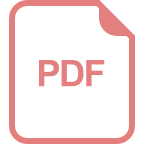