flutter怎么通过路由从别的页面跳转到TabBar和TabBarView里面页面的某一个不特定的页面
时间: 2024-04-13 16:30:47 浏览: 20
要从另一个页面跳转到TabBar和TabBarView中的某个特定页面,您可以在页面之间传递参数,并在TabBarView中使用参数来确定当前应该显示哪个页面。以下是一个示例:
假设您有三个页面:HomePage、TabbedPage和DetailPage。HomePage包含一个按钮,点击该按钮将导航到TabbedPage,并传递一个标识参数用于确定应该显示哪个页面。TabbedPage包含TabBar和TabBarView,每个标签对应一个页面。DetailPage是TabBarView中的某个特定页面。
首先,在HomePage中,您可以使用Navigator.push方法跳转到TabbedPage,并传递一个参数来标识应该显示哪个页面:
```dart
import 'package:flutter/material.dart';
import 'tabbed_page.dart';
class HomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Home'),
),
body: Center(
child: RaisedButton(
child: Text('Go to Tabbed Page'),
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => TabbedPage(selectedIndex: 1)), // 传递参数selectedIndex为1
);
},
),
),
);
}
}
void main() {
runApp(MaterialApp(
home: HomePage(),
));
}
```
然后,在TabbedPage中,您可以通过DefaultTabController和TabBarView来管理标签和相应的页面。通过接收传递的selectedIndex参数来确定初始选中的页面。
```dart
import 'package:flutter/material.dart';
import 'tab_page.dart';
class TabbedPage extends StatelessWidget {
final int selectedIndex;
TabbedPage({this.selectedIndex});
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: 3,
initialIndex: selectedIndex,
child: Scaffold(
appBar: AppBar(
title: Text('Tabbed Page'),
bottom: TabBar(
tabs: [
Tab(text: 'Page 1'),
Tab(text: 'Page 2'),
Tab(text: 'Page 3'),
],
),
),
body: TabBarView(
children: [
TabPage(title: 'Page 1'),
TabPage(title: 'Page 2'),
DetailPage(),
],
),
),
);
}
}
class TabPage extends StatelessWidget {
final String title;
TabPage({this.title});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Text(title),
),
);
}
}
class DetailPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Text('Detail Page'),
),
);
}
}
```
在上面的示例中,我们在TabbedPage中接收selectedIndex参数,并使用initialIndex来确定初始选中的页面。在TabBarView中,我们根据传递的selectedIndex来决定显示哪个页面。
当您从HomePage点击按钮跳转到TabbedPage时,将会显示TabBar和TabBarView,并且特定的页面(例如DetailPage)将会被选中。
希望对您有所帮助!如果有任何其他问题,请随时提问。
相关推荐
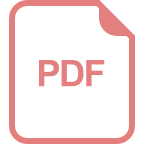
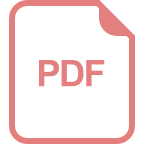
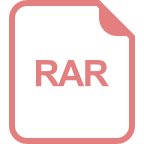
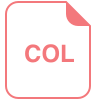
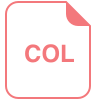












