flutter从其他子页面的跳转到TabBar,TabBarView中某个页面时,不覆盖底部导航栏,详细实现流程代码
时间: 2024-04-15 08:29:06 浏览: 276
要实现从其他子页面跳转到TabBar中的某个页面时,不覆盖底部导航栏,你可以使用Flutter中的IndexedStack组件来管理页面堆栈,并在跳转时设置相关参数以实现你的需求。
首先,在你的TabBar页面中,定义一个变量来存储当前选中的页面索引:
```dart
int currentIndex = 0;
```
然后,在TabBarView中使用IndexedStack来管理页面堆栈:
```dart
IndexedStack(
index: currentIndex,
children: [
// 页面1
// 页面2
// 页面3
],
)
```
接下来,在底部导航栏中,使用BottomNavigationBar来显示导航按钮,并在点击按钮时更新currentIndex的值:
```dart
BottomNavigationBar(
currentIndex: currentIndex,
onTap: (index) {
setState(() {
currentIndex = index;
});
},
items: [
BottomNavigationBarItem(
icon: Icon(Icons.page1),
label: 'Page 1',
),
BottomNavigationBarItem(
icon: Icon(Icons.page2),
label: 'Page 2',
),
BottomNavigationBarItem(
icon: Icon(Icons.page3),
label: 'Page 3',
),
],
)
```
这样,通过点击底部导航栏上的按钮,可以在TabBarView中切换到对应的页面,且底部导航栏始终保持可见。
接下来,在其他子页面中,通过Navigator组件进行跳转到TabBar页面的某个页面时,可以使用Navigator.pushReplacement方法替换当前路由,并将currentIndex参数传递过去:
```dart
Navigator.pushReplacement(
context,
MaterialPageRoute(
builder: (context) => TabBarPage(currentIndex: desiredIndex),
),
);
```
在TabBarPage中,接收currentIndex参数,并在初始化时将其赋值给currentIndex变量:
```dart
class TabBarPage extends StatefulWidget {
final int currentIndex;
TabBarPage({this.currentIndex = 0});
@override
_TabBarPageState createState() => _TabBarPageState();
}
class _TabBarPageState extends State<TabBarPage> {
int currentIndex;
@override
void initState() {
super.initState();
currentIndex = widget.currentIndex;
}
@override
Widget build(BuildContext context) {
return Scaffold(
// TabBar和TabBarView的实现
// ...
);
}
}
```
这样,在从其他子页面跳转到TabBar中的某个页面时,底部导航栏不会被覆盖,并且保持正确的页面索引。
希望以上代码示例能帮到你!如果还有其他问题,请随时提问。
阅读全文
相关推荐
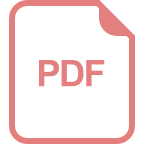
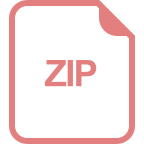
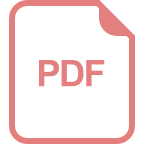





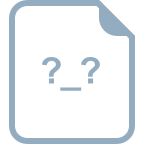
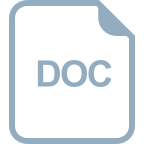
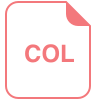
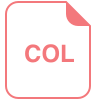

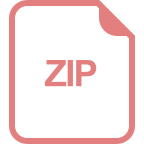
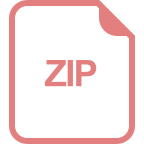
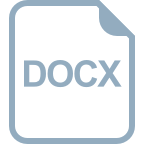
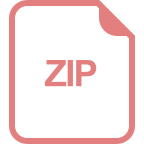
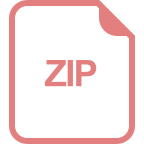