python 同目录下所有jpg图片根据重合部分进行拼接,并保存为新图片
时间: 2024-10-11 19:04:47 浏览: 23
在Python中,我们可以使用PIL(Python Imaging Library)或其更现代的替代品Pillow库来处理图像,以及matplotlib或opencv等库来进行图像操作和比较。要根据重合部分拼接同目录下的jpg图片,你需要完成以下几个步骤:
1. 导入所需的库:
```python
from PIL import Image
import os
```
2. 定义一个函数来计算两张图片的重叠区域:
```python
def get_intersection(img1_path, img2_path):
im1 = Image.open(img1_path)
im2 = Image.open(img2_path)
intersection_area = im1.crop(im1.getbbox()).crop(im2.getbbox())
if not intersection_area:
return None
else:
return intersection_area
```
3. 遍历文件夹,找出所有.jpg文件并尝试拼接:
```python
def merge_images_by_overlap(directory, output_image_path):
images = [img for img in os.listdir(directory) if img.endswith('.jpg')]
merged_image = Image.new('RGB', (0, 0))
for i, img1 in enumerate(images[:-1]):
for img2 in images[i+1:]:
intersection = get_intersection(os.path.join(directory, img1), os.path.join(directory, img2))
# 如果找到重叠,合并到merged_image上
if intersection is not None:
width, height = intersection.size
merged_image.paste(intersection, (merged_image.width, merged_image.height))
merged_image.width += width
merged_image.height = max(merged_image.height, height)
merged_image.save(output_image_path)
```
4. 调用合并函数:
```python
merge_images_by_overlap('/path/to/your/directory', 'output_mosaic.jpg')
```
注意替换`'/path/to/your/directory'`为你实际图片所在的目录。
阅读全文
相关推荐
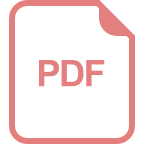
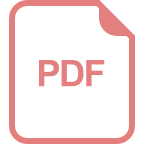
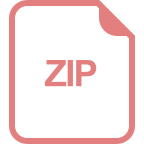
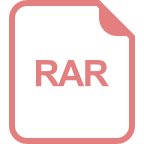
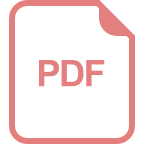
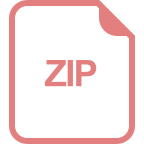
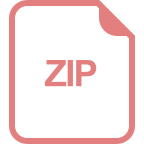
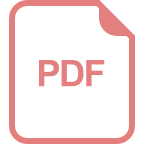
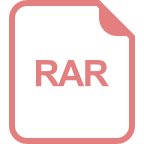
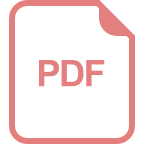
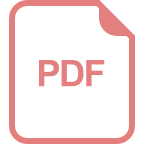
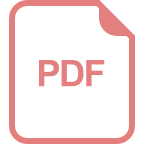
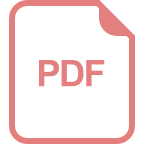
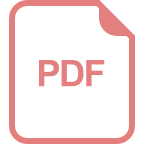
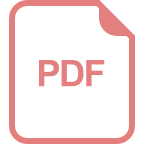
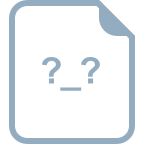
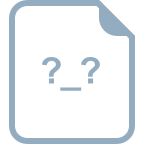