1.先让用户输入一个年份,你判断输出这一年是否是闰年。 2.接着再让用户输入月份,你判断这个数字是否是1-12的有效输入,然后提示重新输入。 3.直到输入了正确月份,然后你判断输出这个月有多少天。
时间: 2024-09-12 15:06:04 浏览: 40
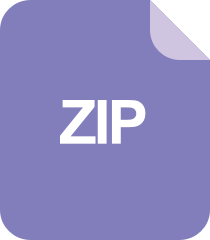
runnian.zip_闰年_闰年判断
好的,我们可以按照步骤设计一个简单的Java程序来实现这些功能:
首先,我们编写一个方法来判断是否是闰年:
```java
private static boolean isLeapYear(int year) {
if (year % 4 == 0) {
if (year % 100 == 0) {
if (year % 400 == 0) {
return true;
}
return false;
}
return true;
}
return false;
}
```
接下来,用户输入年份并检查闰年的部分:
```java
Scanner scanner = new Scanner(System.in);
System.out.print("请输入一个年份:");
int inputYear = scanner.nextInt();
if (!isLeapYear(inputYear)) {
System.out.println(year + " 年不是闰年。");
} else {
System.out.println(year + " 年是闰年。");
}
```
现在,我们添加一个循环来获取有效月份:
```java
while (true) {
System.out.print("请输入月份(1-12):");
int inputMonth = scanner.nextInt();
if (inputMonth >= 1 && inputMonth <= 12) {
break; // 输入有效,跳出循环
}
System.out.println("无效输入,请重新输入!");
}
```
最后,输出对应月份的天数:
```java
switch (inputMonth) {
case 1: // January
case 3: // March
case 5: // May
case 7: // July
case 8: // August
case 10: // October
case 12: // December
System.out.println(inputMonth + " 月有 31 天。");
break;
case 4: // April
case 6: // June
case 9: // September
case 11: // November
System.out.println(inputMonth + " 月有 30 天。");
break;
case 2: // February
if (isLeapYear(inputYear)) {
System.out.println(inputMonth + " 月有 29 天(闰年).");
} else {
System.out.println(inputMonth + " 月有 28 天(平年).");
}
break;
}
// 关闭扫描器
scanner.close();
```
现在你的程序就能满足这三个要求了。
阅读全文
相关推荐
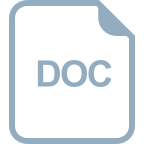
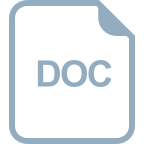
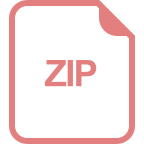





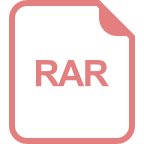
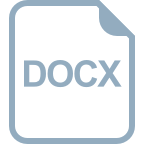
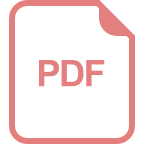






