vue2 openlayers
时间: 2023-09-24 19:00:30 浏览: 178
Vue是一种用户界面开发框架,而OpenLayers是一个专门用于地理信息系统(GIS)开发的JavaScript库。通过将这两者结合使用,我们可以开发出功能强大的地理信息应用程序。
Vue2 OpenLayers是指使用Vue2框架来构建一个集成OpenLayers功能的应用程序。Vue2提供了一种声明式的语法和组件化的开发方式,能够更轻松地管理应用程序的状态和界面。而OpenLayers则提供了一系列用于加载、显示、交互、分析和操作地图数据的功能。
使用Vue2 OpenLayers的好处包括:
1. 组件化开发:我们可以将地图、图层、标记、工具栏等功能封装为Vue组件,方便复用和维护。
2. 响应式设计:Vue2的响应式机制可以自动追踪数据变化,并更新相应的界面,使地图应用能够实时地反映数据的变化。
3. 灵活的数据绑定:Vue2支持双向数据绑定,可以将地图数据与界面元素进行绑定,用户操作地图时,数据会相应地更新。
4. 强大的交互功能:OpenLayers提供了丰富的交互功能,如地图缩放、平移、标记绘制、要素选择等,能够满足各种地图应用的需求。
5. 异步加载数据:Vue2支持异步组件加载,可以通过按需加载地图数据,提高应用程序的加载性能。
综上所述,Vue2 OpenLayers是一种利用Vue2框架和OpenLayers库开发地理信息应用程序的技术组合,能够提供灵活、高效和交互性强的地图应用。
相关问题
vue2 openlayers 点击标记
要在Vue2中添加点击标记的功能,您需要遵循以下步骤:
1. 首先,您需要引入OpenLayers库和Vue2:
```html
<!-- 引入OpenLayers库 -->
<script src="https://cdn.jsdelivr.net/npm/ol@6.5.0/dist/ol.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/ol@6.5.0/dist/ol.css" />
<!-- 引入Vue2 -->
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
```
2. 然后,您需要在Vue组件中创建地图和标记:
```html
<template>
<div>
<div ref="map" style="height: 400px;"></div>
</div>
</template>
<script>
export default {
mounted() {
// 创建地图
const map = new ol.Map({
target: this.$refs.map,
layers: [
new ol.layer.Tile({
source: new ol.source.OSM(),
}),
],
view: new ol.View({
center: ol.proj.fromLonLat([116.3975, 39.9082]),
zoom: 12,
}),
});
// 创建标记
const marker = new ol.Feature({
geometry: new ol.geom.Point(ol.proj.fromLonLat([116.3975, 39.9082])),
});
const markerLayer = new ol.layer.Vector({
source: new ol.source.Vector({
features: [marker],
}),
style: new ol.style.Style({
image: new ol.style.Icon({
anchor: [0.5, 1],
src: 'https://cdn.rawgit.com/openlayers/ol-mapbox-style/master/sprite.png',
scale: 0.05,
imgSize: [512, 512],
crossOrigin: 'anonymous',
offset: [0, 512],
}),
}),
});
map.addLayer(markerLayer);
},
};
</script>
```
3. 最后,您需要添加标记点击事件的处理程序:
```html
<template>
<div>
<div ref="map" style="height: 400px;"></div>
</div>
</template>
<script>
export default {
mounted() {
// 创建地图
const map = new ol.Map({
target: this.$refs.map,
layers: [
new ol.layer.Tile({
source: new ol.source.OSM(),
}),
],
view: new ol.View({
center: ol.proj.fromLonLat([116.3975, 39.9082]),
zoom: 12,
}),
});
// 创建标记
const marker = new ol.Feature({
geometry: new ol.geom.Point(ol.proj.fromLonLat([116.3975, 39.9082])),
});
const markerLayer = new ol.layer.Vector({
source: new ol.source.Vector({
features: [marker],
}),
style: new ol.style.Style({
image: new ol.style.Icon({
anchor: [0.5, 1],
src: 'https://cdn.rawgit.com/openlayers/ol-mapbox-style/master/sprite.png',
scale: 0.05,
imgSize: [512, 512],
crossOrigin: 'anonymous',
offset: [0, 512],
}),
}),
});
map.addLayer(markerLayer);
// 监听标记点击事件
map.on('click', (event) => {
const feature = map.forEachFeatureAtPixel(event.pixel, (feature) => {
return feature;
});
if (feature === marker) {
alert('您点击了标记!');
}
});
},
};
</script>
```
在这个例子中,我们使用`map.on('click', ...)`来监听地图点击事件。然后,我们使用`map.forEachFeatureAtPixel(event.pixel, ...)`来获取被点击的标记。如果被点击的标记是我们创建的那个标记,就弹出一个提示框。
这就是在Vue2中添加点击标记的全部步骤。
vue2 openlayers 廊坊市中聚合点 点击标注信息弹窗的代码
以下是一个简单的示例代码,用于在Vue2中使用OpenLayers 6来显示一个地图,添加一个聚合点,并在点击聚合点时显示相关的标注信息弹窗:
```html
<template>
<div id="map"></div>
</template>
<script>
import 'ol/ol.css'
import { Map, View } from 'ol'
import Point from 'ol/geom/Point'
import Feature from 'ol/Feature'
import { Tile as TileLayer, Vector as VectorLayer } from 'ol/layer'
import { fromLonLat } from 'ol/proj'
import { Vector as VectorSource } from 'ol/source'
import { Cluster } from 'ol/source'
import Overlay from 'ol/Overlay'
export default {
name: 'MapComponent',
data() {
return {
map: null,
popup: null
}
},
mounted() {
this.initMap()
},
methods: {
initMap() {
// 创建地图
this.map = new Map({
target: 'map',
layers: [
new TileLayer({
source: new XYZ({
url: 'https://{a-c}.tile.openstreetmap.org/{z}/{x}/{y}.png'
})
})
],
view: new View({
center: fromLonLat([116.7, 39.5]),
zoom: 10
})
})
// 创建聚合点
const clusterSource = new Cluster({
distance: 40,
source: new VectorSource({
features: [
new Feature(new Point(fromLonLat([116.718, 39.523]))),
new Feature(new Point(fromLonLat([116.729, 39.504]))),
new Feature(new Point(fromLonLat([116.752, 39.506]))),
new Feature(new Point(fromLonLat([116.745, 39.521]))),
new Feature(new Point(fromLonLat([116.738, 39.53]))),
new Feature(new Point(fromLonLat([116.707, 39.534]))),
new Feature(new Point(fromLonLat([116.698, 39.526]))),
new Feature(new Point(fromLonLat([116.686, 39.517])))
]
})
})
const clusterLayer = new VectorLayer({
source: clusterSource
})
this.map.addLayer(clusterLayer)
// 创建弹窗
const popupContainer = document.createElement('div')
popupContainer.setAttribute('id', 'popup')
popupContainer.setAttribute('class', 'ol-popup')
document.body.appendChild(popupContainer)
this.popup = new Overlay({
element: popupContainer,
autoPan: true,
autoPanAnimation: {
duration: 250
}
})
this.map.addOverlay(this.popup)
// 监听聚合点点击事件
this.map.on('click', (event) => {
const feature = this.map.forEachFeatureAtPixel(event.pixel, (feature) => {
return feature
})
if (feature && feature.get('features').length > 1) {
const coordinates = feature.getGeometry().getCoordinates()
const count = feature.get('features').length
const content = `
<p>共有${count}个标注点</p>
`
this.popup.setPosition(coordinates)
this.popup.getElement().innerHTML = content
}
})
}
}
}
</script>
<style>
.ol-popup {
position: absolute;
background-color: white;
box-shadow: 0 1px 4px rgba(0, 0, 0, 0.2);
padding: 15px;
border-radius: 10px;
border: 1px solid #cccccc;
bottom: 12px;
left: -50px;
min-width: 200px;
}
</style>
```
该示例代码创建了一个地图,并在其中添加了一个聚合点,每个聚合点包含多个标注点。当用户点击聚合点时,会弹出一个标注信息弹窗,显示该聚合点中包含的标注点数量。你可以根据自己的需要修改代码中的坐标、弹窗内容等信息。
阅读全文
相关推荐
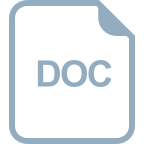
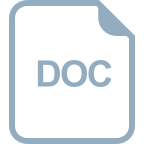
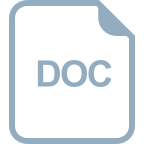

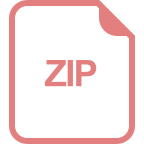
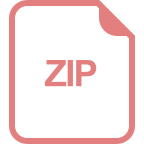










