pygame 设置窗口坐标原点
时间: 2024-12-26 15:24:45 浏览: 5
### 如何在 Pygame 中设置窗口坐标系的原点位置
为了使坐标系的原点位于窗口中心,在 Pygame 中可以采用特定的方法来实现这一需求。默认情况下,Pygame 的坐标系是以画布的左上角作为原点 (0, 0),其中 x 轴正方向指向右侧而 y 轴正方向指向下侧[^3]。
当目标是在编写游戏时调整原点至屏幕中央,则可以通过应用平移变换的方式达成目的。具体来说,可以在绘制对象之前先计算出相对于新原点的位置偏移量,并据此更新待渲染物体的实际显示位置。对于每次绘图操作而言,这意味着要基于当前视窗尺寸动态确定新的中心点坐标并相应地调整所有图形元素的位置数据[^1]。
下面给出一段 Python 代码示例用于展示如何通过上述思路实现在 Pygame 中更改坐标系统的起点:
```python
import pygame
import sys
def main():
# 初始化 Pygame 库
pygame.init()
width, height = 800, 600
screen_center_x, screen_center_y = width / 2, height / 2
# 创建一个指定大小的游戏窗口
window_surface = pygame.display.set_mode((width, height))
clock = pygame.time.Clock()
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
# 清屏
window_surface.fill(pygame.Color('white'))
# 绘制一些测试形状到屏幕上
draw_test_shapes(window_surface, screen_center_x, screen_center_y)
# 刷新整个屏幕的内容
pygame.display.flip()
clock.tick(30)
def draw_test_shapes(surface, center_x, center_y):
"""Draw shapes with origin at the given coordinates."""
red_color = pygame.Color('red')
blue_color = pygame.Color('blue')
# 圆形:以传入的新原点为中心绘制
circle_radius = 50
pygame.draw.circle(
surface,
red_color,
(center_x, center_y), # 新的圆心位置
circle_radius
)
# 正方形:同样考虑到了新的原点位置进行位移处理
square_size = 100
top_left_corner_of_square = (
int(center_x - square_size/2),
int(center_y - square_size/2)
)
pygame.draw.rect(
surface,
blue_color,
(*top_left_corner_of_square, square_size, square_size)
)
if __name__ == '__main__':
main()
```
此程序创建了一个白色背景的游戏窗口,并在其内部居中位置分别用红色和蓝色绘制了一圈圆形以及一个正方形。这里的关键在于 `draw_test_shapes` 函数接收了两个额外参数——即代表新原点坐标的 `center_x` 和 `center_y` ——从而允许我们轻松地将任何几何体放置于期望之处。
阅读全文
相关推荐
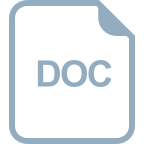
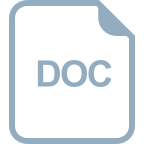
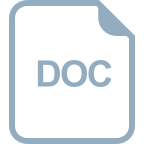

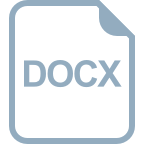
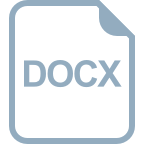
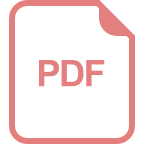
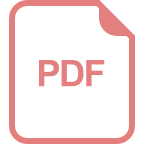
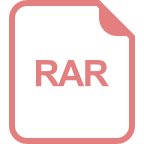
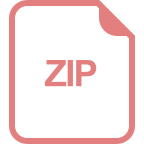
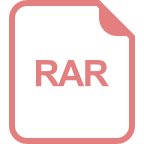
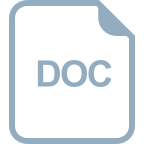
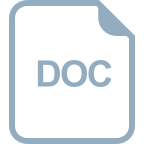
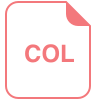
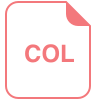
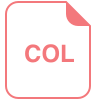
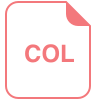

