编写一个C++程序,用户输入一个整数N,其取值范围为(-2147483647, 2147483647),然后将该数转换为其原码表示(包括一位符号位和31位数值位),并以十进制的形式显示输出。如何实现这一功能?
时间: 2024-12-11 08:18:52 浏览: 12
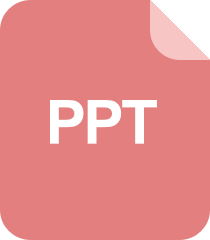
枚举类型-C++程序设计
在C++中,你可以通过使用位运算符来实现这个功能。下面是一个简单的程序示例,它首先获取用户的输入,然后将其转换为原码形式,并打印出来:
```cpp
#include <iostream>
using namespace std;
// Function to convert integer to its one's complement representation
int onesComplement(int num) {
return num ^ ((1 << 31) - 1); // Bitwise XOR with all set bits (32nd bit as sign)
}
int main() {
int N;
// Get user input
cout << "Enter an integer in the range (-2147483647, 2147483647): ";
cin >> N;
// Check if the number is within the valid range
if(N < -2147483648 || N > 2147483647) {
cerr << "Number out of range. Please enter a value between -2147483647 and 2147483647." << endl;
return 1;
}
// Convert the number to one's complement
int onesComp = onesComplement(N);
// Since we use one's complement for signed integers, the most significant bit indicates the sign
bool isNegative = (onesComp & (1 << 31)) != 0; // Check the sign by testing the MSB
// Shift the number right to get rid of the sign bit
onesComp &= ~(1 << 31);
// Print the result in decimal format
cout << (isNegative ? "-" : "") << "Original: " << N << ", One's Complement: " << onesComp << endl;
return 0;
}
```
在这个程序中,我们首先计算了输入数字的原码表示(对于有符号整数,这通常就是它的补码,因为计算机内部使用二进制补码表示),然后检查最右边的一位作为符号位。如果结果是负数,我们就加上负号;然后右移一位置换出原始数值部分。
阅读全文
相关推荐
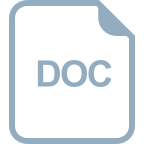
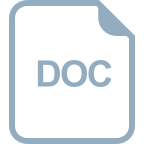
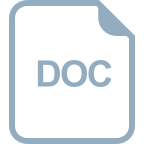
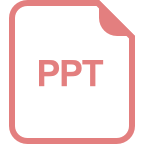
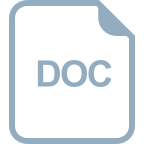
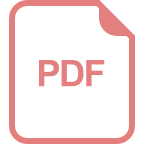
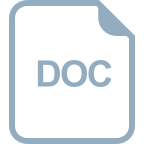
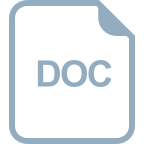
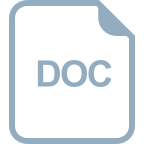
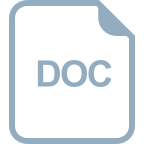
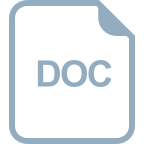
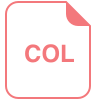
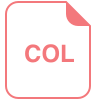


