举例strcpy的程序
时间: 2024-04-30 21:15:16 浏览: 77
strcpy是C语言中的一个字符串复制函数,用于将一个字符串的内容复制到另一个字符串中。下面是一个使用strcpy函数的示例程序:
```c
#include <stdio.h>
#include <string.h>
int main() {
char source[] = "Hello, World!"; // 源字符串
char destination[20]; // 目标字符串
strcpy(destination, source); // 复制源字符串到目标字符串
printf("源字符串: %s\n", source);
printf("目标字符串: %s\n", destination);
return 0;
}
```
在这个示例程序中,我们首先定义了一个源字符串`source`和一个目标字符串`destination`。然后使用`strcpy`函数将源字符串的内容复制到目标字符串中。最后,我们打印出源字符串和目标字符串的内容。
运行该程序,输出结果为:
```
源字符串: Hello, World!
目标字符串: Hello, World!
```
这说明`strcpy`函数成功地将源字符串的内容复制到了目标字符串中。
相关问题
你这个举例是两个应用程序,请举一个应用程序与一个内核模块之间共享大量数据的例子
好的,下面是一个应用程序与一个内核模块之间共享大量数据的例子:
1. 内核模块定义一个共享内存结构体和相应的操作函数:
```c
#include <linux/init.h>
#include <linux/module.h>
#include <linux/kernel.h>
#include <linux/fs.h>
#include <linux/uaccess.h>
#include <linux/slab.h>
#include <linux/mutex.h>
#define SHM_SIZE 4096
struct my_shm {
int num;
char buf[SHM_SIZE];
struct mutex lock;
};
static struct my_shm* shm;
static int my_shm_open(struct inode* inode, struct file* filp)
{
return 0;
}
static int my_shm_release(struct inode* inode, struct file* filp)
{
return 0;
}
static ssize_t my_shm_read(struct file* filp, char __user* buf, size_t count, loff_t* f_pos)
{
ssize_t ret = 0;
if (*f_pos < sizeof(struct my_shm)) {
ret = min(count, sizeof(struct my_shm) - *f_pos);
if (copy_to_user(buf, (char*)shm + *f_pos, ret) != 0) {
ret = -EFAULT;
}
*f_pos += ret;
}
return ret;
}
static ssize_t my_shm_write(struct file* filp, const char __user* buf, size_t count, loff_t* f_pos)
{
ssize_t ret = 0;
if (*f_pos < sizeof(struct my_shm)) {
ret = min(count, sizeof(struct my_shm) - *f_pos);
if (copy_from_user((char*)shm + *f_pos, buf, ret) != 0) {
ret = -EFAULT;
}
*f_pos += ret;
}
return ret;
}
static struct file_operations my_shm_fops = {
.owner = THIS_MODULE,
.open = my_shm_open,
.release = my_shm_release,
.read = my_shm_read,
.write = my_shm_write,
};
static int __init my_shm_init(void)
{
shm = (struct my_shm*)kmalloc(sizeof(struct my_shm), GFP_KERNEL);
if (shm == NULL) {
return -ENOMEM;
}
memset(shm, 0, sizeof(struct my_shm));
mutex_init(&shm->lock);
if (register_chrdev(0, "my_shm", &my_shm_fops) < 0) {
kfree(shm);
return -EFAULT;
}
return 0;
}
static void __exit my_shm_exit(void)
{
unregister_chrdev(0, "my_shm");
kfree(shm);
}
MODULE_LICENSE("GPL");
module_init(my_shm_init);
module_exit(my_shm_exit);
```
2. 应用程序使用共享内存结构体和相应的操作函数进行数据交换:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <string.h>
#include <sys/mman.h>
#define SHM_PATH "/dev/my_shm"
struct my_shm {
int num;
char buf[4096];
};
int main()
{
int fd = open(SHM_PATH, O_RDWR);
if (fd < 0) {
perror("open");
return -1;
}
struct my_shm* shm = (struct my_shm*)mmap(NULL, sizeof(struct my_shm), PROT_READ | PROT_WRITE, MAP_SHARED, fd, 0);
if (shm == MAP_FAILED) {
perror("mmap");
close(fd);
return -1;
}
strcpy(shm->buf, "Hello, kernel!");
printf("The kernel says: %s\n", shm->buf);
munmap(shm, sizeof(struct my_shm));
close(fd);
return 0;
}
```
在这个例子中,内核模块定义了一个名为my_shm的字符设备,并实现了相关的文件操作函数,如读、写、打开和关闭等。在应用程序中,通过打开/dev/my_shm设备并使用mmap系统调用将其映射到内存中,应用程序可以访问共享内存结构体shm并进行数据读写操作。需要注意的是,为了保证数据的一致性和安全性,内核模块中使用了mutex互斥锁来同步访问共享内存。
程序编码的过程是怎样的,能否举例说明
### 赫夫曼编码过程概述
赫夫曼编码是一种用于无损数据压缩的前缀编码方法。其主要步骤包括:
1. **统计频率**:计算每个字符出现的频率。
2. **构建赫夫曼树**:根据频率构建一棵二叉树,频率越低的字符离根节点越远。
3. **生成编码**:从叶子节点到根节点逆向生成每个字符的编码。
4. **编码文本**:使用生成的编码对原始文本进行编码。
### 具体实现步骤
#### 1. 统计频率
首先,程序会要求用户输入字符及其对应的权重(频率)。例如:
```c
printf("请输入字符数n:");
scanf("%d", &n);
getchar();
for(i=1; i<=n; i++) {
printf("输入字符和权值:");
scanf("%c%d", &HT[i].elem, &HT[i].weight);
getchar();
}
```
#### 2. 构建赫夫曼树
通过 `HuffmanCoding` 函数构建赫夫曼树:
```c
void HuffmanCoding(int n) {
int i, m, s1, s2, start, c, f;
char *cd;
m = 2 * n - 1;
for(i = n + 1; i <= m; ++i) {
HT[i].elem = '0';
HT[i].weight = HT[i].parent = HT[i].lchild = HT[i].rchild = 0;
}
for(i = n + 1; i <= m; ++i) {
Select(i - 1, &s1, &s2);
HT[s1].parent = i;
HT[s2].parent = i;
HT[i].lchild = s1;
HT[i].rchild = s2;
HT[i].weight = HT[s1].weight + HT[s2].weight;
}
// 生成编码
HC = (HuffmanCode)malloc((n + 1) * sizeof(char *));
cd = (char *)malloc(n * sizeof(char));
cd[n - 1] = '\0';
for(i = 1; i <= n; ++i) {
start = n - 1;
for(c = i, f = HT[i].parent; f != 0; c = f, f = HT[f].parent) {
if(HT[f].lchild == c) cd[--start] = '0';
else cd[--start] = '1';
}
HC[i] = (char *)malloc((n - start) * sizeof(char));
strcpy(HC[i], &cd[start]);
}
free(cd);
return;
}
```
#### 3. 生成编码
在构建赫夫曼树后,从叶子节点到根节点逆向生成每个字符的编码。
#### 4. 编码文本
通过 `Encoding` 函数将输入的文本转换为赫夫曼编码:
```c
void Encoding() {
int i, j, all;
char temp[1000], code[10000];
printf("请输入需要编码的句子:");
gets(temp);
code[0] = '\0';
FILE *FToBeTranP = NULL;
if(NULL == (FToBeTranP = fopen("C:\\Users\\a1370\\Desktop\\ToBeTran.txt", "w")))
printf("打开 ToBeTran.txt 文件失败!\n");
else
fputs(temp, FToBeTranP);
fclose(FToBeTranP);
TempLen = strlen(temp);
for(i = 0; i < TempLen; i++) {
all = 0;
for(j = 1; j <= n; j++) {
if(temp[i] == HT[j].elem) {
strcat(code, HC[j]);
all = 1;
}
}
if(all == 0)
printf("句子中有不匹配的字符!!!");
}
code_num = strlen(code);
printf("句子的编码为:\n%s\n", code);
FILE *FCodeFileP = NULL;
if(NULL == (FCodeFileP = fopen("C:\\Users\\a1370\\Desktop\\CodeFile.txt", "w")))
printf("打开 CodeFile.txt 文件失败!\n");
else
fputs(code, FCodeFileP);
fclose(FCodeFileP);
printf("内容已经被输入CodeFile.txt!\n");
return;
}
```
### 示例
假设输入字符及频率如下:
- A: 45
- B: 13
- C: 12
- D: 16
- E: 9
- F: 5
#### 构建赫夫曼树
1. 初始化节点:
```
Node 1: A (45)
Node 2: B (13)
Node 3: C (12)
Node 4: D (16)
Node 5: E (9)
Node 6: F (5)
```
2. 选择最小的两个节点合并:
- 合并 F (5) 和 E (9),生成新节点 7 (14)
- 合并 C (12) 和 7 (14),生成新节点 8 (26)
- 合并 B (13) 和 8 (26),生成新节点 9 (39)
- 合并 D (16) 和 9 (39),生成新节点 10 (55)
- 合并 A (45) 和 10 (55),生成新节点 11 (100)
#### 生成编码
从叶子节点到根节点逆向生成编码:
- A: 0
- B: 101
- C: 100
- D: 111
- E: 1101
- F: 1100
#### 编码文本
假设输入文本为 "ABCD":
- A -> 0
- B -> 101
- C -> 100
- D -> 111
最终编码结果为:
```
0101100111
```
该编码结果会被保存到 `CodeFile.txt` 中。
阅读全文
相关推荐
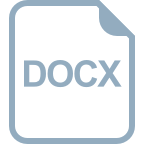
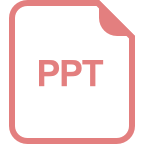
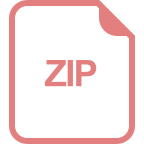
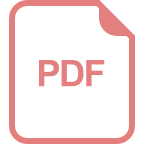
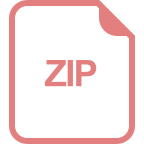
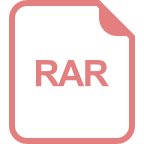
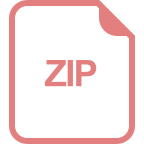
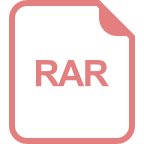
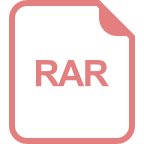
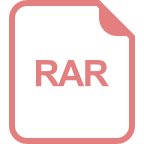
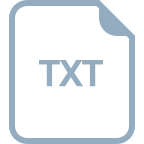
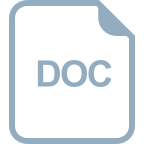

