c语言通过文件获取顺序栈
时间: 2024-10-06 15:03:26 浏览: 28
在C语言中,通过文件实现顺序存储栈,通常涉及到两个步骤:首先,将数据结构存入文件;其次,从文件中读取数据重构栈。这里假设我们使用整数作为元素类型。
1. **向文件中存放栈**:
- 定义一个栈结构体,包含一个数组用于存储元素和栈顶指针(top)。
```c
#define MAX_SIZE 100
typedef struct {
int stack[MAX_SIZE];
int top;
} Stack;
```
- 当向栈中添加元素并达到最大容量时,可以考虑先保存到文件。例如,使用`fopen()`打开文件,然后用`fprintf()`写入栈顶元素及其数量。
```c
void save_to_file(Stack *s, char *filename) {
FILE *file = fopen(filename, "w");
if (file == NULL) {
printf("Failed to open file.\n");
return;
}
fprintf(file, "%d\n", s->top);
for (int i = 0; i < s->top; i++) {
fprintf(file, "%d ", s->stack[i]);
}
fclose(file);
}
```
2. **从文件中加载栈**:
- 从文件读取数据,创建空栈,然后按照读取的顺序填充。
```c
Stack load_from_file(char *filename) {
Stack s;
s.top = 0;
FILE *file = fopen(filename, "r");
if (file == NULL) {
printf("Failed to open file.\n");
return s;
}
int num_elements;
fscanf(file, "%d", &num_elements);
while (num_elements--) {
s.stack[s.top++] = fscanf(file, "%d");
}
fclose(file);
return s;
}
```
阅读全文
相关推荐
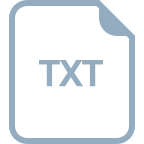
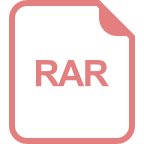
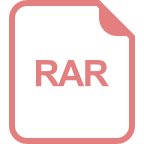

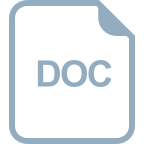
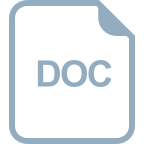
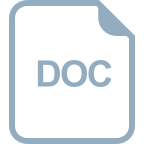
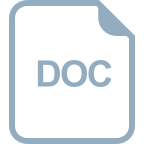
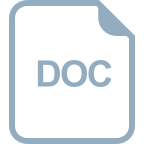
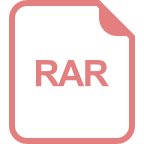
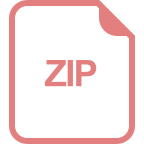
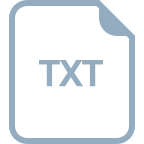
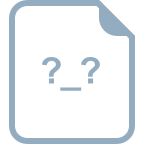
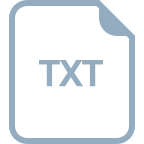
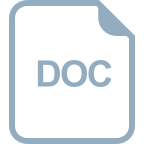
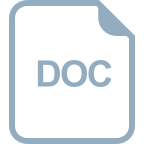
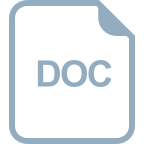
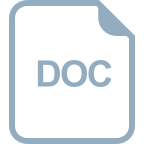