编程实现图像变暗,变亮,降低对比度,gamma变换,应具有可视化输出的代码
时间: 2024-09-09 12:08:26 浏览: 66
在Python中,我们可以使用PIL(Pillow)库来实现图像的处理操作,包括变暗、变亮、降低对比度和伽马变换。这里是一个简单的示例:
```python
from PIL import Image, ImageEnhance
def adjust_brightness(image_path, factor):
# 加载图片
img = Image.open(image_path)
# 创建亮度增强器
enhancer = ImageEnhance.Brightness(img)
# 变亮或变暗图片
if factor > 0:
adjusted_img = enhancer.enhance(factor) # 亮度增加
else:
adjusted_img = enhancer.enhance(abs(factor)) # 亮度减小
# 可视化输出
adjusted_img.show()
def adjust_contrast(image_path, factor):
img = Image.open(image_path)
enhancer = ImageEnhance.Contrast(img)
adjusted_img = enhancer.enhance(factor)
adjusted_img.show()
def gamma_correction(image_path, gamma):
img = Image.open(image_path).convert('RGB')
gray_img = img.convert('L') # 转为灰度图像以便于进行伽玛校正
inv_gamma = 1.0 / gamma
table = [((i / 255.0)**inv_gamma * 255.0) for i in range(256)]
lookup_table = bytes([table[i] for i in range(256)])
new_img = Image.frombytes('L', img.size, lookup_table)
final_img = Image.merge("RGB", (new_img, new_img, new_img))
final_img.show()
# 示例应用
# 调整亮度,设factor=0.5表示变暗50%
adjust_brightness("input.jpg", 0.5)
# 调整对比度,设factor=1.5表示增强对比度
adjust_contrast("input.jpg", 1.5)
# 伽玛变换,设gamma=0.8(小于1会使图像变暗)
gamma_correction("input.jpg", 0.8)
阅读全文
相关推荐






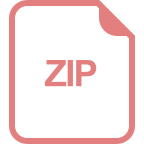










