pyqt5 QTableWidget 关闭右键
时间: 2024-02-05 09:05:16 浏览: 31
你可以通过以下方法来关闭pyqt5中的QTableWidget的右键菜单:
1. 在创建QTableWidget时,设置setContextMenuPolicy为Qt.CustomContextMenu,然后连接customContextMenuRequested信号到一个槽函数,该槽函数用于关闭右键菜单。
```python
tableWidget.setContextMenuPolicy(Qt.CustomContextMenu)
tableWidget.customContextMenuRequested.connect(closeContextMenu)
```
2. 在槽函数closeContextMenu中,使用close方法关闭右键菜单。
```python
def closeContextMenu():
tableWidget.closePersistentEditor(tableWidget.currentItem())
```
相关问题
pyqt5 QTableWidget 设置右键后不取消
可以通过设置QTableWidget的上下文菜单策略来实现右键点击后不取消的效果。具体步骤如下:
1. 创建一个自定义的QTableWidget子类,并重写其contextMenuEvent方法,用于处理右键菜单事件。
2. 在contextMenuEvent方法中创建并显示自定义的右键菜单。
3. 在右键菜单的每个动作中,设置setCheckable(True)以使其可选中,并设置setChecked(True)以保持选中状态。
4. 在每个动作的triggered信号中,添加相应的处理逻辑。
下面是一个示例代码:
```python
from PyQt5.QtWidgets import QTableWidget, QMenu, QAction
class CustomTableWidget(QTableWidget):
def __init__(self, parent=None):
super().__init__(parent)
self.setContextMenuPolicy(Qt.CustomContextMenu)
self.customContextMenuRequested.connect(self.showContextMenu)
def showContextMenu(self, pos):
menu = QMenu(self)
action1 = QAction("Action 1", self)
action1.setCheckable(True)
action1.setChecked(True)
action1.triggered.connect(self.action1Clicked)
menu.addAction(action1)
action2 = QAction("Action 2", self)
action2.setCheckable(True)
action2.setChecked(True)
action2.triggered.connect(self.action2Clicked)
menu.addAction(action2)
menu.exec_(self.mapToGlobal(pos))
def action1Clicked(self):
# 处理Action 1的逻辑
pass
def action2Clicked(self):
# 处理Action 2的逻辑
pass
```
你可以将上述代码添加到你的PyQt5应用程序中,并使用CustomTableWidget替换原来的QTableWidget,以实现右键点击后不取消的效果。
PyQt5 QTableWidget右键表头可以弹出菜单
可以通过继承QTableWidget类并重写mousePressEvent方法来实现右键菜单的弹出。
首先,创建一个新类并继承QTableWidget:
```python
class MyTableWidget(QTableWidget):
def __init__(self, parent=None):
super().__init__(parent)
```
然后,重写mousePressEvent方法:
```python
class MyTableWidget(QTableWidget):
def __init__(self, parent=None):
super().__init__(parent)
def mousePressEvent(self, event):
if event.button() == Qt.RightButton:
pos = event.pos()
if self.horizontalHeader().rect().contains(pos):
menu = QMenu(self)
menu.addAction("Action 1")
menu.addAction("Action 2")
menu.exec_(self.horizontalHeader().mapToGlobal(pos))
```
在mousePressEvent方法中,我们首先检查鼠标按下的是右键,然后检查鼠标的位置是否在水平表头内。如果是,我们创建一个QMenu并添加一些动作,然后在鼠标位置处显示菜单。
最后,我们需要将表格部件设置为我们新创建的表格类:
```python
table = MyTableWidget()
```
完整的示例代码:
```python
from PyQt5.QtWidgets import QApplication, QMainWindow, QTableWidget, QMenu
from PyQt5.QtCore import Qt
class MyTableWidget(QTableWidget):
def __init__(self, parent=None):
super().__init__(parent)
def mousePressEvent(self, event):
if event.button() == Qt.RightButton:
pos = event.pos()
if self.horizontalHeader().rect().contains(pos):
menu = QMenu(self)
menu.addAction("Action 1")
menu.addAction("Action 2")
menu.exec_(self.horizontalHeader().mapToGlobal(pos))
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
table = MyTableWidget()
table.setRowCount(4)
table.setColumnCount(3)
self.setCentralWidget(table)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.show()
app.exec_()
```
相关推荐
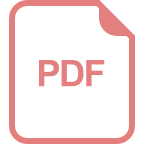
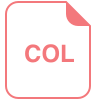
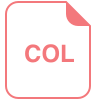
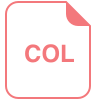
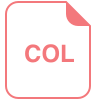
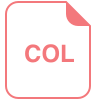







