pyqt5 qtablewidget
时间: 2023-04-25 14:01:07 浏览: 171
PyQt5 是一个 Python 的 GUI 库,QTableWidget 是 PyQt5 中用于显示表格数据的类。它可以显示多行多列的数据,并支持编辑、选择等常用操作。使用 PyQt5 和 QTableWidget 可以轻松地创建带有表格界面的应用程序。
相关问题
PyQt5 QTableWidget
PyQt5中的QTableWidget是一个用于显示和编辑表格数据的控件。它是Qt框架中QTableWidget类的Python绑定,提供了丰富的功能和灵活性,适用于各种表格数据展示和操作需求。以下是QTableWidget的一些主要特点和功能:
1. **表格创建和设置**:
- 创建QTableWidget对象时,可以指定行数和列数。
- 可以设置表格的行列标签和表格的整体属性。
2. **单元格操作**:
- 可以通过行和列的索引访问和修改单元格的内容。
- 支持设置单元格的文本、对齐方式、字体、颜色等属性。
- 可以设置单元格的编辑权限,例如只读或可编辑。
3. **行和列操作**:
- 可以插入、删除和移动行和列。
- 可以设置行高和列宽。
4. **信号和槽机制**:
- 支持多种信号,如单元格点击、单元格编辑等,方便实现交互功能。
5. **样式和外观**:
- 可以自定义表格的样式,包括网格线、背景色等。
以下是一个简单的示例代码,展示了如何使用QTableWidget:
```python
import sys
from PyQt5.QtWidgets import QApplication, QTableWidget, QTableWidgetItem, QVBoxLayout, QWidget
class TableExample(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle('QTableWidget Example')
self.setGeometry(100, 100, 600, 400)
layout = QVBoxLayout()
table = QTableWidget()
table.setRowCount(3)
table.setColumnCount(3)
table.setHorizontalHeaderLabels(['Header 1', 'Header 2', 'Header 3'])
table.setItem(0, 0, QTableWidgetItem('Cell (0,0)'))
table.setItem(0, 1, QTableWidgetItem('Cell (0,1)'))
table.setItem(0, 2, QTableWidgetItem('Cell (0,2)'))
table.setItem(1, 0, QTableWidgetItem('Cell (1,0)'))
table.setItem(1, 1, QTableWidgetItem('Cell (1,1)'))
table.setItem(1, 2, QTableWidgetItem('Cell (1,2)'))
table.setItem(2, 0, QTableWidgetItem('Cell (2,0)'))
table.setItem(2, 1, QTableWidgetItem('Cell (2,1)'))
table.setItem(2, 2, QTableWidgetItem('Cell (2,2)'))
layout.addWidget(table)
self.setLayout(layout)
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = TableExample()
ex.show()
sys.exit(app.exec_())
```
这个示例创建了一个简单的表格,包含3行3列,并设置了表头和单元格内容。
pyqt5 qtablewidgetitem
QTableWidgetItem is a class in PyQt5 that is used to create items for the QTableWidget. It is used to set and retrieve data from the cells of the QTableWidget.
Some of the important methods of the QTableWidgetItem class are:
- setText(): Sets the text of the item.
- text(): Returns the text of the item.
- setData(): Sets the data of the item.
- data(): Returns the data of the item.
- setBackground(): Sets the background color of the item.
- foreground(): Returns the foreground color of the item.
Example:
```
from PyQt5.QtWidgets import QApplication, QMainWindow, QTableWidget, QTableWidgetItem
import sys
class Table(QMainWindow):
def __init__(self):
super().__init__()
# Creating a table widget object
self.table = QTableWidget(self)
# Adding rows and columns to the table
self.table.setRowCount(4)
self.table.setColumnCount(3)
# Adding items to the table
for i in range(4):
for j in range(3):
item = QTableWidgetItem("Item " + str(i) + " " + str(j))
self.table.setItem(i, j, item)
# Setting the table widget as the central widget
self.setCentralWidget(self.table)
# Creating the application
app = QApplication(sys.argv)
# Creating the window
window = Table()
window.show()
# Running the application
sys.exit(app.exec_())
```
In this example, we create a QTableWidget with 4 rows and 3 columns. We add items to the table using the QTableWidgetItem class. The setText() method is used to set the text of the item. The setItem() method is used to add the item to the table. Finally, we set the table widget as the central widget of the window.
阅读全文
相关推荐
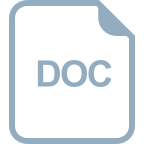
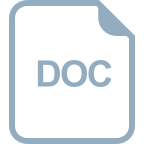
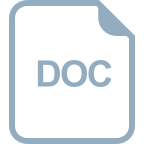













