delphi7 对汉字加密和解密的例子
时间: 2024-09-27 11:10:32 浏览: 35
Delphi 7,作为 Borland 公司的一款古老的版本,主要用于Windows应用程序开发,其中可以利用其强大的VCL库进行文本处理,包括对汉字进行加密和解密。这里提供一个简单的示例,展示如何使用常见的DES(Data Encryption Standard)算法进行加解密:
```delphi
uses CryptoAPI; // 导入CryptoAPI单元,包含DES加密函数
// 加密函数
function EncryptString(const PlainText: string): string;
var
Key: array[0..7] of byte; // DES key (假设长度为8字节)
I: Integer;
begin
Key := ... // 实际上需要从安全来源获取密钥
Result := '';
for I := Low(Key) to High(Key) do
Result := Result + Hex(Key[I]) + ':'; // 字符串拼接每个字节的十六进制表示
Result := Result + '||'; // 结束符
// 使用CryptProtectMemory确保明文内存安全,然后加密
var Buffer: PByte; Size: Integer;
Size := Length(PlainText);
Buffer := AllocateMemory(Size + 4); // 额外添加4字节用于存储长度信息
Move(PChar(PlainText), Buffer^, Size);
Inc(Buffer^, Size); // 跳过明文
try
CryptProtectMemory(Buffer, Size+4, True); // 设置保护标志
Result := Cryptoapi.CryptEncrypt(
nil, // NULL handle for default provider (DES)
False, // No padding
@Buffer^,
Size,
nil, // Output buffer - not used for encryption
0, // Output length - ignored
DES,
0); // Use the standard DES cipher
// 更新结果字符串,包含长度信息
Result := Result + IntToHex(Size div 16, 2) + ':' +
IntToHex((Size mod 16) div 1, 2) + ':' +
Copy(PChar(Buffer)^, Size div 16 * 2, Size mod 16);
finally
FreeMemory(Buffer);
end;
end;
// 解密函数
function DecryptString(const EncryptedText: string): string;
var
Key: array[0..7] of byte; // 同样的DES key
LengthLow, LengthHigh: Byte;
DecryptedBuffer: PByteArray; // 存储解密后的内存缓冲区
begin
Result := '';
// 从加密字符串提取长度信息
LengthLow := StrToInt(ExtractWord(EncryptedText, 0));
LengthHigh := ExtractWord(EncryptedText, 2);
// 分配解密缓冲区并读取数据
DecryptedBuffer := AllocateMemory(LengthHigh * 2 + 1);
ReadStr(DecryptedBuffer^, EncryptedText, Pos(':', EncryptedText));
try
// 进行解密
Result := '';
CryptUnprotectMemory(@DecryptedBuffer^, LengthHigh * 2 + 1, True);
Result := System.UniStringBuilder.Create(
PChar(DecryptedBuffer)^, LengthHigh * 2).ToString();
finally
FreeMemory(DecryptedBuffer);
end;
end;
阅读全文
相关推荐
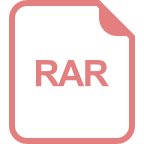
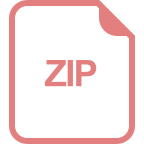
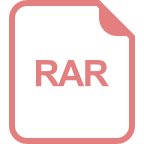















