jquery中siblings的功能
时间: 2024-06-17 10:06:40 浏览: 221
在jQuery中,siblings()方法返回与指定元素相邻的所有同级元素。这个方法可以用来遍历某个元素的所有兄弟元素,或者通过选择器来筛选出特定的兄弟元素。
siblings()方法有以下几种用法:
1. siblings():返回当前元素的所有同级元素。
2. siblings(selector):返回当前元素的特定类型的同级元素。
3. siblings(filter):返回当前元素的通过筛选函数过滤后的同级元素。
例如,如果我们有以下HTML结构:
```
<ul>
<li>Item 1</li>
<li class="highlight">Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
<li class="highlight">Item 5</li>
</ul>
```
我们可以使用以下jQuery代码来获取highlight类别的li元素的同级元素:
```
$(".highlight").siblings()
```
这将返回两个li元素(Item 1和Item 3)。
相关问题
jQuery实现购买功能
jQuery 可以用于实现购买功能的前端交互部分,但是后端数据处理和数据库存储等需要使用其他技术实现。下面是一个简单的 jQuery 实现购买功能的示例:
HTML 代码:
```
<!-- 商品列表 -->
<div class="item">
<h3>商品1</h3>
<p>价格:100元</p>
<button class="buy-btn" data-id="1">购买</button>
</div>
<div class="item">
<h3>商品2</h3>
<p>价格:200元</p>
<button class="buy-btn" data-id="2">购买</button>
</div>
<!-- 购物车 -->
<div class="cart">
<h3>购物车</h3>
<ul class="cart-list"></ul>
<p>总价:<span class="total-price">0</span>元</p>
</div>
```
jQuery 代码:
```
$(function() {
// 购买按钮点击事件
$('.buy-btn').click(function() {
var id = $(this).data('id'); // 获取商品ID
var name = $(this).siblings('h3').text(); // 获取商品名称
var price = parseInt($(this).siblings('p').text().replace('价格:', '').replace('元', '')); // 获取商品价格
// 添加到购物车
var item = '<li data-id="' + id + '">' + name + ' - ' + price + '元 <button class="remove-btn">删除</button></li>';
$('.cart-list').append(item);
// 更新总价
var totalPrice = parseInt($('.total-price').text());
$('.total-price').text(totalPrice + price);
});
// 删除按钮点击事件
$('.cart-list').on('click', '.remove-btn', function() {
var price = parseInt($(this).parent().text().replace('元 删除', '').replace(' - ', ''));
$(this).parent().remove();
// 更新总价
var totalPrice = parseInt($('.total-price').text());
$('.total-price').text(totalPrice - price);
});
});
```
上面的代码实现了点击购买按钮将商品添加到购物车,点击删除按钮从购物车中删除商品,并更新购物车总价。需要注意的是,这里只是一个简单的示例,实际项目中需要更完善的购买流程和数据处理机制。
用jQuery实现Apple商城购物车功能
以下是一个简单的使用 jQuery 实现 Apple 商城购物车功能的示例:
HTML 代码:
```html
<div id="cart">
<h2>购物车</h2>
<ul id="cart-items"></ul>
<p>总价:<span id="total-price">0</span> 元</p>
</div>
<div class="product">
<h2>产品名称</h2>
<p>价格:100 元</p>
<button class="add-to-cart">加入购物车</button>
</div>
```
jQuery 代码:
```javascript
$(document).ready(function() {
var cartItems = [];
var totalPrice = 0;
$('.add-to-cart').click(function() {
var productName = $(this).siblings('h2').text();
var productPrice = parseInt($(this).siblings('p').text().replace('价格:', '').replace(' 元', ''));
var item = { name: productName, price: productPrice };
cartItems.push(item);
totalPrice += productPrice;
$('#cart-items').append('<li>' + productName + ' - ' + productPrice + ' 元</li>');
$('#total-price').text(totalPrice);
});
});
```
在上面的示例中,我们首先定义了一个空的 `cartItems` 数组和 `totalPrice` 变量来存储购物车中的商品和总价。然后,我们使用 jQuery 的 `click()` 函数来监听加入购物车按钮的点击事件。在点击事件处理程序中,我们使用 jQuery 的 `siblings()` 函数来获取产品名称和价格,并将它们存储在一个对象中。接着,我们将该对象添加到 `cartItems` 数组中,并将商品价格加到 `totalPrice` 变量中。最后,我们使用 jQuery 的 `append()` 函数将商品信息添加到购物车列表中,并更新总价显示。
阅读全文
相关推荐
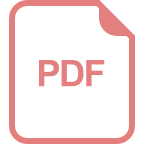
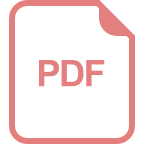
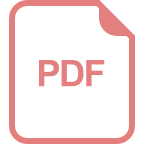













