python 实现一个类似悬浮球的按钮,悬浮球可以鼠标移动位置,可以右键关闭。 点击悬浮球可以打开一个新的带折线图的界面,再次点击悬浮球可以隐藏该界面。
时间: 2024-10-20 08:08:53 浏览: 27
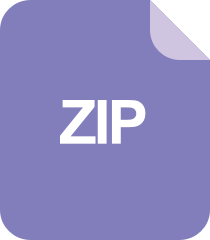
基于pyside6开发的windows平台悬浮球工具python源码(含项目说明+超详细注释).zip

在Python中,特别是使用PyQt5或Kivy这样的GUI库,你可以轻松地实现一个浮动按钮功能。这里我会给出一个基本的例子,使用PyQt5库,因为它的事件处理机制非常适合这类需求。
首先,你需要安装PyQt5,如果你还没有安装,可以通过pip安装:
```bash
pip install PyQt5
```
然后,这里是一个简单的例子:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QPushButton, QVBoxLayout, QGraphicsView, QGraphicsScene, QMenu, QAction
from PyQt5.QtGui import QPainter, QColor, QPen
from PyQt5.QtCore import Qt, QPoint
class HoverButton(QPushButton):
def __init__(self, parent=None):
super().__init__(parent)
self.setFixedSize(50, 50) # 悬浮球尺寸
self.setCursor(Qt.OpenHandCursor) # 鼠标悬停显示手形光标
self.is_visible = False
self.scene = QGraphicsScene()
self.view = QGraphicsView(self.scene)
def mouseMoveEvent(self, event): # 鼠标移动时调整位置
if not self.is_visible:
self.move(event.globalPos())
def mousePressEvent(self, event): # 右键关闭
if event.button() == Qt.RightButton and self.is_visible:
self.hide()
def show_graph(self):
if self.is_visible:
return
self.scene.addRect(0, 0, 100, 100, color=QColor('blue')) # 添加折线图元素
self.view.setScene(self.scene)
self.is_visible = True
self.show()
def hide_graph(self):
if self.is_visible:
self.scene.clear()
self.view.setScene(None)
self.is_visible = False
self.hide()
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.hover_button = HoverButton()
layout = QVBoxLayout()
layout.addWidget(self.hover_button)
widget = QWidget()
widget.setLayout(layout)
self.setCentralWidget(widget)
# 右键菜单
menu = QMenu()
action = QAction("Close Graph", self)
action.triggered.connect(self.hover_button.hide_graph)
menu.addAction(action)
self.hover_button.setContextMenu(menu)
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
在这个例子中,`HoverButton`类继承自QPushButton,添加了鼠标移动和右键关闭的功能,并实现了打开/隐藏折线图的功能。当右键点击按钮时,会弹出一个菜单,选择"Close Graph"动作会隐藏图形界面。
阅读全文
相关推荐
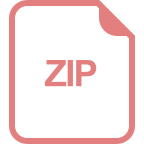
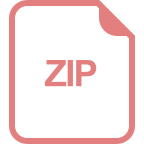
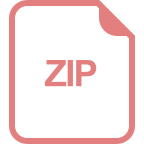
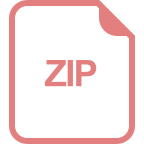
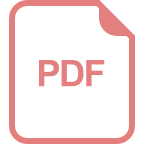
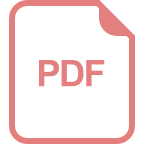
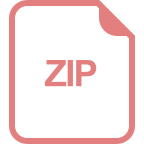
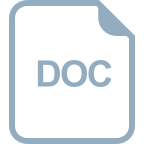
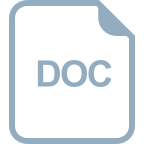
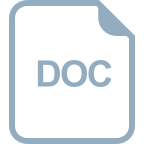



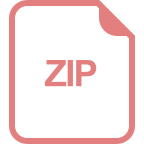
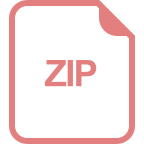