1.Write a Python program to create a singly linked list, append some items and iterate through the list.
时间: 2024-09-30 20:14:56 浏览: 20
在Python中创建一个单向链表并进行添加和遍历,你可以按照以下步骤操作:
```python
# 定义链表节点类
class Node:
def __init__(self, data=None):
self.data = data
self.next = None
# 创建链表类
class LinkedList:
def __init__(self):
self.head = None
# 添加元素到链表尾部
def append(self, data):
if not self.head:
self.head = Node(data)
else:
current = self.head
while current.next:
current = current.next
current.next = Node(data)
# 遍历链表
def display(self):
elements = []
current = self.head
while current:
elements.append(current.data)
current = current.next
print("Elements in the linked list:", elements)
# 测试链表
my_list = LinkedList()
my_list.append(1)
my_list.append(2)
my_list.append(3)
my_list.display() # 输出: Elements in the linked list: [1, 2, 3]
```
在这个程序中,我们首先定义了一个`Node`类表示链表的每个节点,包含数据和指向下一个节点的引用。然后,我们在`LinkedList`类中实现了链表的头部管理以及添加元素(`append`方法)和显示链表内容(`display`方法)。最后,我们创建一个实例并演示了如何添加和遍历元素。
相关推荐
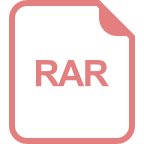
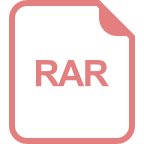
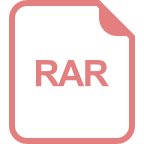
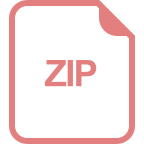
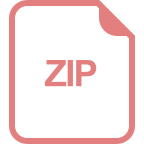
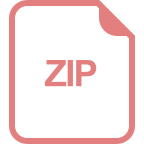
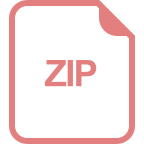









