Define Linked-List Write java program to perform a)Declare Node b)Add item in the Node c)Display the entire List d)Add Item at Start e)Add Item at End
时间: 2024-02-18 11:04:45 浏览: 106
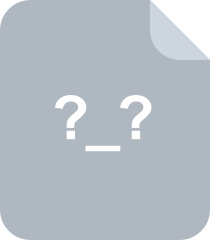
Java实现LinkedList
Sure, here is an example Java program that demonstrates the basic operations of a singly linked-list:
```java
public class LinkedListExample {
class Node {
int data;
Node next;
public Node(int data) {
this.data = data;
this.next = null;
}
}
Node head;
public void add(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
public void addAtStart(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
newNode.next = head;
head = newNode;
}
}
public void addAtEnd(int data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
public void display() {
Node current = head;
if (head == null) {
System.out.println("List is empty.");
} else {
while (current != null) {
System.out.print(current.data + " -> ");
current = current.next;
}
System.out.println("null");
}
}
public static void main(String[] args) {
LinkedListExample list = new LinkedListExample();
// add items to the list
list.add(5);
list.add(10);
list.add(15);
// display the list
System.out.println("Original List:");
list.display();
// add item at start
list.addAtStart(1);
System.out.println("List after adding item at start:");
list.display();
// add item at end
list.addAtEnd(20);
System.out.println("List after adding item at end:");
list.display();
}
}
```
Here is a brief explanation of the program:
- The `Node` class represents a single node in the linked-list. It has two attributes: `data` to store the value of the node and `next` to store a reference to the next node in the list.
- The `LinkedListExample` class represents the linked-list itself. It has a `head` attribute which stores a reference to the first node in the list.
- The `add` method adds a new node to the end of the list. It first creates a new node with the given data. If the list is empty (i.e. `head` is `null`), it sets the new node as the head. Otherwise, it traverses the list until it reaches the last node and sets the `next` attribute of the last node to the new node.
- The `addAtStart` method adds a new node to the beginning of the list. It first creates a new node with the given data. If the list is empty (i.e. `head` is `null`), it sets the new node as the head. Otherwise, it sets the `next` attribute of the new node to the current head and sets the new node as the head.
- The `addAtEnd` method adds a new node to the end of the list, similar to the `add` method.
- The `display` method traverses the list and prints the value of each node. If the list is empty, it prints a message indicating that the list is empty.
- The main method creates a new `LinkedListExample` object, adds some items to the list, and calls the `display` method to print the original list. Then, it adds an item at the start of the list and calls `display` again to print the updated list. Finally, it adds an item at the end of the list and calls `display` again to print the final list.
阅读全文
相关推荐
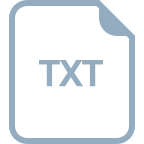



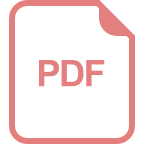
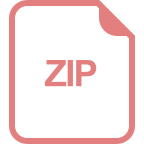
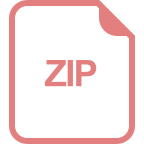
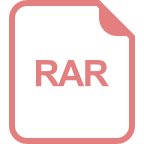
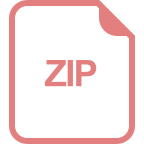
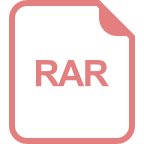
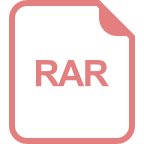
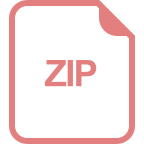
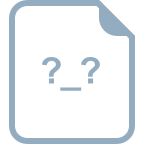
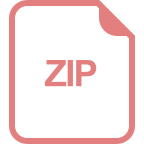
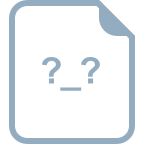
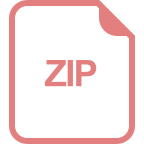
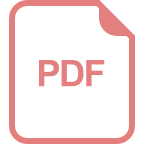