Write a program that reverses a list using a for loop and creates a new list with the reversed elements
时间: 2024-10-26 09:16:11 浏览: 6
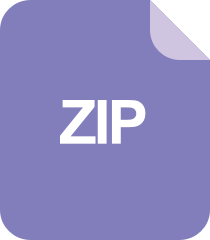
Takes a string and reverses it.
To create a program that reverses a list using a `for` loop, you first need to define a list and then iterate through it in reverse order to populate a new list. Here's how you can do it:
```csharp
// Define the original list
List<int> originalList = new List<int>() { 1, 2, 3, 4, 5 };
// Create a new empty list for the reversed elements
List<int> reversedList = new List<int>();
// Use a for loop to reverse the elements
for (int i = originalList.Count - 1; i >= 0; i--)
{
// Add the current element from the original list to the reversed list
reversedList.Add(originalList[i]);
}
// Print the reversed list
Console.WriteLine($"Reversed list: {reversedList}");
```
In this example, we start from the last index (`originalList.Count - 1`) of the `originalList`, decrementing `i` until we reach 0. Each iteration adds the corresponding element from the `originalList` to the `reversedList`.
阅读全文
相关推荐
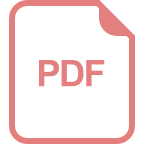
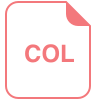
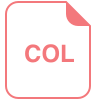













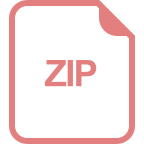
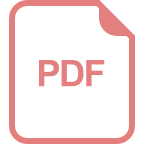
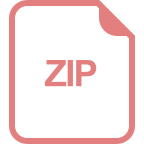