: Write a Python function named "reverse_string" that takes a string as input and returns the reverse of the input string.
时间: 2024-03-22 07:39:11 浏览: 121
Sure! Here is the Python function named "reverse_string" that takes a string as input and returns the reverse of the input string:
```python
def reverse_string(input_string):
return input_string[::-1]
```
This function uses Python slicing to reverse the input string. The `[::]` notation means to start at the end of the string and step backwards by one character until the beginning of the string is reached. This effectively reverses the string.
相关问题
Question 3.1: Write a Python function named "is_even" that takes an integer as input and returns True if the number is even, and False otherwise. Question 3.2: Write a Python function named "reverse_string" that takes a string as input and returns the reverse of the input string. Question 3.3: Write a Python function named "find_max" that takes a list of numbers as input and returns the maximum value from the list. Question 3.4: Write a Python function named "validate_password" that takes a string as input and checks if it is a valid password. A valid password should meet the following criteria: • It must be at least 8 characters long. • It must contain at least one uppercase letter, one lowercase letter, and one dig
Answer 3.1:
Here is the Python function "is_even" that takes an integer as input and returns True if the number is even, and False otherwise:
```python
def is_even(num):
if num % 2 == 0:
return True
else:
return False
```
Answer 3.2:
Here is the Python function "reverse_string" that takes a string as input and returns the reverse of the input string:
```python
def reverse_string(input_str):
return input_str[::-1]
```
Answer 3.3:
Here is the Python function "find_max" that takes a list of numbers as input and returns the maximum value from the list:
```python
def find_max(nums):
max_num = nums[0]
for num in nums:
if num > max_num:
max_num = num
return max_num
```
Answer 3.4:
Here is the Python function "validate_password" that takes a string as input and checks if it is a valid password:
```python
def validate_password(password):
if len(password) < 8:
return False
has_uppercase = False
has_lowercase = False
has_digit = False
for char in password:
if char.isupper():
has_uppercase = True
elif char.islower():
has_lowercase = True
elif char.isdigit():
has_digit = True
if has_uppercase and has_lowercase and has_digit:
return True
else:
return False
```
Write a JavaScript function named reverse, which is used as an input parameter of the “sort" function, l.e., sori (reverse), to implement the following requirements: Input: A line of text, using prompt. Output: The words of the input text, in alphabetical reverse order. Note: you are only required to write the reverse function, rather than the entire html code
Here is the JavaScript function named `reverse` that can be used as an input parameter of the `sort` function:
```
function reverse(a, b) {
if (a < b) {
return 1;
} else if (a > b) {
return -1;
} else {
return 0;
}
}
```
To use this `reverse` function with the `sort` function to output the words of an input text in alphabetical reverse order, you can follow these steps:
1. Use the `prompt` function to get a line of text from the user and store it in a variable:
```
let inputText = prompt("Enter a line of text:");
```
2. Split the input text into an array of words using the `split` function:
```
let words = inputText.split(" ");
```
3. Sort the words in alphabetical order using the `sort` function and pass the `reverse` function as an input parameter:
```
words.sort(reverse);
```
4. Join the sorted words back into a string using the `join` function:
```
let outputText = words.join(" ");
```
5. Output the sorted words in alphabetical reverse order to the user:
```
alert(outputText);
```
Putting it all together, here's the full code:
```
function reverse(a, b) {
if (a < b) {
return 1;
} else if (a > b) {
return -1;
} else {
return 0;
}
}
let inputText = prompt("Enter a line of text:");
let words = inputText.split(" ");
words.sort(reverse);
let outputText = words.join(" ");
alert(outputText);
```
阅读全文
相关推荐
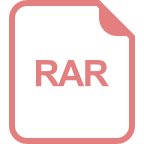
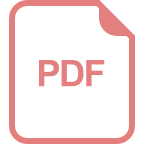
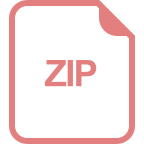
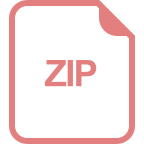
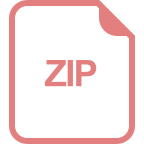
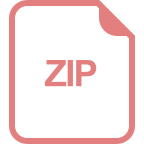
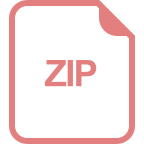
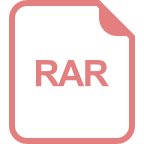
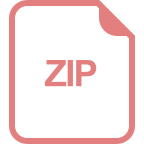
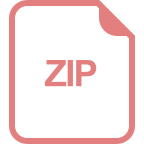
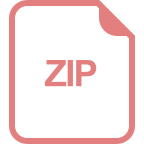



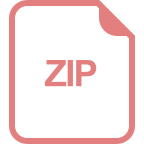